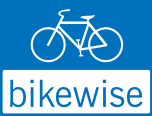
BikeWise
Sports & FitnessExploring the Bikewise API with JavaScript
Introduction
APIs (Application Programming Interfaces) are essential tools for developers to build applications that can access and manipulate data from various sources. One such API that we will be exploring today is the Bikewise API, which provides information about bike accidents and stolen bikes across the globe. In this article, we will explore the API, its functionalities and how we can use it with JavaScript.
Getting started
To get started, we need to head to the Bikewise API website and sign up to obtain an API key. Once we have our API key, we can use it to access the API resources and start making API calls.
API Endpoints
The Bikewise API has two endpoints for accessing its resources. The first endpoint is https://bikewise.org:443/api/v2/
, which provides information about bike accidents. The second endpoint is https://bikewise.org:443/api/v2/incidents/
, which provides information about stolen bikes.
HTTP Requests and Responses
Using these endpoints, we can make HTTP requests to the server and obtain responses in JSON format. The requests we can make are GET, POST, PUT and DELETE. The response we get will depend on the type of request we make.
API Examples
Let's look at some examples of how we can use the Bikewise API with JavaScript. For these examples, we will be using the Axios library to make HTTP requests.
Get the total number of bike accidents
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const TOTAL_ACCIDENTS_ENDPOINT = 'https://bikewise.org:443/api/v2/incidents/count';
axios.get(`${TOTAL_ACCIDENTS_ENDPOINT}?proximity_square=100`)
.then(response => {
console.log(`Total bike accidents: ${response.data.incident_count}`);
})
.catch(error => {
console.log(error);
});
Get bike accidents within a certain proximity
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const PROXIMITY_ENDPOINT = 'https://bikewise.org:443/api/v2/incidents';
axios.get(`${PROXIMITY_ENDPOINT}?proximity=Berlin&proximity_square=100`)
.then(response => {
console.log(response.data.incidents);
})
.catch(error => {
console.log(error);
});
Get a specific bike accident
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const ACCIDENT_ENDPOINT = 'https://bikewise.org:443/api/v2/incidents';
axios.get(`${ACCIDENT_ENDPOINT}/20522`)
.then(response => {
console.log(response.data.incident);
})
.catch(error => {
console.log(error);
});
Get the total number of stolen bikes
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const TOTAL_STOLEN_BIKES_ENDPOINT = 'https://bikewise.org:443/api/v2/incidents/count';
axios.get(`${TOTAL_STOLEN_BIKES_ENDPOINT}?incident_type=theft`)
.then(response => {
console.log(`Total stolen bikes: ${response.data.incident_count}`);
})
.catch(error => {
console.log(error);
});
Get a specific stolen bike
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const STOLEN_BIKE_ENDPOINT = 'https://bikewise.org:443/api/v2/incidents';
axios.get(`${STOLEN_BIKE_ENDPOINT}/1234`)
.then(response => {
console.log(response.data.incident);
})
.catch(error => {
console.log(error);
});
Conclusion
In this article, we explored the Bikewise API and how we can use it with JavaScript to obtain information about bike accidents and stolen bikes. We looked at the API endpoints, HTTP requests and responses, and provided examples of how we can interact with the API. With this knowledge, we can now build applications that can access and manipulate the data provided by the Bikewise API.