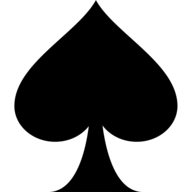
Deck of Cards
Games & ComicsAutomate your Card Games with DeckofCardsAPI
DeckofCardsAPI is an open source API that allows for the automation of card games. Developers can easily integrate this API into their code to fetch, shuffle, draw, and manipulate decks of cards. In this blog post, we will explore the possible API example codes in JavaScript.
Getting Started
Before we start coding, let's take a look at the documentation provided on the website http://deckofcardsapi.com/. The website provides detailed information about the API, including endpoints, query parameters, and responses.
Fetching and Shuffling a New Deck
To fetch and shuffle a new deck, we can use the fetch()
function to send a GET request to the https://deckofcardsapi.com/api/deck/new/shuffle/
endpoint. This will return a JSON object containing the deck ID, which we can use to perform further actions.
fetch('https://deckofcardsapi.com/api/deck/new/shuffle/')
.then(response => response.json())
.then(data => console.log(data));
Drawing a Card from a Deck
To draw a card from a deck, we need to know the deck ID obtained from the previous step. We can then use the fetch()
function to send a GET request to the https://deckofcardsapi.com/api/deck/{deck_id}/draw/
endpoint by replacing {deck_id}
with the actual deck ID. This will return a JSON object containing information about the drawn card.
const deckId = '<<deck_id>>'; // replace with actual deck ID
fetch(`https://deckofcardsapi.com/api/deck/${deckId}/draw/`)
.then(response => response.json())
.then(data => console.log(data));
Drawing Multiple Cards from a Deck
Sometimes, we need multiple cards from a deck for a game. We can use the count
query parameter to specify the number of cards we want to draw. The fetch()
function will then send a GET request to https://deckofcardsapi.com/api/deck/{deck_id}/draw/?count={count}
with the actual deck ID and the desired count. This will return a JSON object containing information about the drawn cards.
const deckId = '<<deck_id>>'; // replace with actual deck ID
const count = 5; // number of cards to draw
fetch(`https://deckofcardsapi.com/api/deck/${deckId}/draw/?count=${count}`)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
DeckofCardsAPI is a powerful tool that allows developers to automate card games on the web. With the above code examples, developers can get started with drawing, shuffling, and manipulating decks of cards. Have fun building your web-based card games with DeckofCardsAPI!