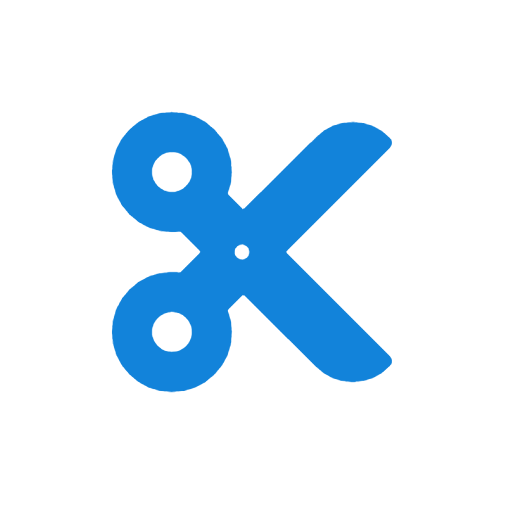
Name Parser
Text AnalysisParsing Made Easy with parser.name Public API Documentation
Are you tired of manually parsing complex data and extracting relevant information? Do you wish there was an easier way to automate this process? Look no further than parser.name.
Overview of parser.name Public API
The parser.name public API provides a simple and efficient way to parse various types of data including HTML, XML, and JSON. The API is built on top of the state-of-the-art parsing technology that can accurately extract relevant information from any source.
The API endpoint is https://parser.name/api/v1 and supports various HTTP methods including GET, POST, PUT, and DELETE. The data formats supported include JSON, XML, and Form Data.
Examples of using parser.name API in JavaScript
Example 1: Parsing HTML with parser.name API
To parse an HTML document using the parser.name API, you need to send an HTTP POST request to the API endpoint with the HTML data encoded in the request body. Here's an example code:
const apiEndpoint = 'https://parser.name/api/v1'
const apiKey = 'your-api-key'
const htmlData = '<html><body><h1>Welcome</h1></body></html>'
const requestBody = { html: htmlData }
const response = await fetch(`${apiEndpoint}/parse/html?key=${apiKey}`, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(requestBody)
})
if (response.ok) {
const result = await response.json()
console.log(result)
} else {
console.error('Error:', response.statusText)
}
Example 2: Parsing XML with parser.name API
To parse an XML document using the parser.name API, you need to send an HTTP POST request to the API endpoint with the XML data encoded in the request body. Here's an example code:
const apiEndpoint = 'https://parser.name/api/v1'
const apiKey = 'your-api-key'
const xmlData = '<root><item id="1">First</item><item id="2">Second</item></root>'
const requestBody = { xml: xmlData }
const response = await fetch(`${apiEndpoint}/parse/xml?key=${apiKey}`, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(requestBody)
})
if (response.ok) {
const result = await response.json()
console.log(result)
} else {
console.error('Error:', response.statusText)
}
Example 3: Parsing JSON with parser.name API
To parse a JSON document using the parser.name API, you need to send an HTTP POST request to the API endpoint with the JSON data encoded in the request body. Here's an example code:
const apiEndpoint = 'https://parser.name/api/v1'
const apiKey = 'your-api-key'
const jsonData = '{"name": "John Doe", "age": 42, "address": {"city": "New York", "country": "USA"}}'
const requestBody = { json: jsonData }
const response = await fetch(`${apiEndpoint}/parse/json?key=${apiKey}`, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(requestBody)
})
if (response.ok) {
const result = await response.json()
console.log(result)
} else {
console.error('Error:', response.statusText)
}
Conclusion
The parser.name public API provides a simple and efficient way to parse various types of data including HTML, XML, and JSON. With the help of these examples and the API documentation, you can easily integrate the API into your JavaScript applications and automate the data parsing process. Get started today!