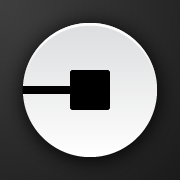
Uber
TransportationExploring the Uber API with JavaScript
Are you looking to integrate the Uber API into your application or website? The first step is getting familiar with the available endpoints and how to make requests to them.
Luckily, the Uber API has a comprehensive documentation website that outlines all of the available endpoints, query parameters, and response formats. Let's dive into it and explore how we can use JavaScript to make API requests.
Setup
Before we begin, make sure to create an Uber developer account and register your application. You'll receive a unique client ID and secret that you'll need to use to authenticate your requests.
In this example, we'll be using Axios to make HTTP requests to the Uber API from JavaScript.
First, install Axios via npm:
npm install axios
Then, import it into your script:
const axios = require('axios');
Example API Requests
Let's take a look at a few example API requests using JavaScript and the Axios library.
Authentication
Before we can make any API requests, we need to authenticate with our client ID and secret.
const clientID = '[YOUR CLIENT ID]';
const clientSecret = '[YOUR CLIENT SECRET]';
axios({
method: 'POST',
url: 'https://login.uber.com/oauth/v2/token',
headers: {
'content-type': 'application/x-www-form-urlencoded'
},
params: {
'client_id': clientID,
'client_secret': clientSecret,
'grant_type': 'client_credentials'
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error.response.data));
This request will return an access token that we can use to make subsequent API requests.
Products
To retrieve a list of available Uber products in a specific city, we can use the /v1.2/products
endpoint.
const accessToken = '[YOUR ACCESS TOKEN]';
const latitude = 37.7752315;
const longitude = -122.418075;
axios({
method: 'GET',
url: 'https://api.uber.com/v1.2/products',
headers: {
'Authorization': `Bearer ${accessToken}`
},
params: {
'latitude': latitude,
'longitude': longitude
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error.response.data));
This request will return a list of available Uber products in the specified location.
Price Estimates
To retrieve price estimates for a specific Uber product, we can use the /v1.2/estimates/price
endpoint.
const accessToken = '[YOUR ACCESS TOKEN]';
const startLatitude = 37.7752315;
const startLongitude = -122.418075;
const endLatitude = 37.787654;
const endLongitude = -122.4027605;
axios({
method: 'GET',
url: 'https://api.uber.com/v1.2/estimates/price',
headers: {
'Authorization': `Bearer ${accessToken}`
},
params: {
'start_latitude': startLatitude,
'start_longitude': startLongitude,
'end_latitude': endLatitude,
'end_longitude': endLongitude
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error.response.data));
This request will return a list of price estimates for the specified Uber product, based on the starting and ending locations.
Conclusion
With the help of the Uber API documentation and a few lines of JavaScript code, we can easily integrate Uber services into our applications and websites. Happy coding!