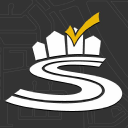
US Street Address
Data ValidationUsing the US Street API from SmartyStreets with JavaScript
SmartyStreets is a provider of address verification services, and their US Street API is a powerful tool that can help you validate and enhance the accuracy of your US address data. In this blog post, we're going to take a closer look at how to use this API with JavaScript.
Setting up the API Key
Before we can start using the US Street API, we need to first get an API key. You can sign up for a free trial of the API on the SmartyStreets website. Once you have your API key, you can start using the API.
Making API Calls
There are a number of different API endpoints that you can use with the US Street API. In this post, we'll focus on the "Street Address" endpoint, which is used to validate and standardize street addresses.
To call the API, we first need to make an HTTP request to the API endpoint using the fetch() function in JavaScript. Here's an example code snippet that shows how to make an API request to the SmartyStreets US Street API using JavaScript:
fetch('https://us-street.api.smartystreets.com/street-address', {
method: 'POST',
body: JSON.stringify([{
street: '1600 Amphitheatre Parkway',
city: 'Mountain View',
state: 'CA'
}]),
headers: {
'Content-Type': 'application/json',
'X-Include-Invalid': 'true',
'auth-id': 'YOUR_AUTH_ID',
'auth-token': 'YOUR_AUTH_TOKEN'
}
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(err => {
console.log(err);
});
In this example, we're making a POST request to the US Street API endpoint with a JSON payload that contains the address information we want to validate and standardize. We're also including the necessary headers to authenticate our API request using our SmartyStreets API key.
Handling API Responses
When we make an API request to the US Street API, we'll receive a JSON response from the server. This response will contain information about the address that we queried, as well as any errors or warnings that may have occurred.
To handle these responses in our JavaScript code, we can use the .then() and .catch() methods on our fetch() promise. In our example code above, we're logging the entire response to the console using console.log(). However, you can modify this code to handle the response data in whatever way makes the most sense for your application.
Conclusion
The SmartyStreets US Street API is a powerful tool for validating and standardizing US street addresses. With a few lines of JavaScript code and your API key in hand, you can easily integrate this API into your application and improve the quality of your address data.