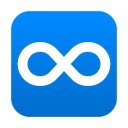
Dynalist API
Documents & ProductivityExploring Dynalist's Public API
Dynalist is a popular note-taking and productivity app that allows users to organize information in a hierarchical format. Dynalist provides a public API that developers can use to build their own integrations or apps on top of Dynalist.
The API documentation is available at apidocs.dynalist.io, and it covers a wide range of API endpoints that allow developers to perform various operations on their Dynalist documents and items.
Getting Started
To begin using the Dynalist API, you first need to obtain a developer API key. You can get your API key by creating a new app on the Dynalist Developer Console.
Once you have your API key, you can start making API requests using JavaScript. Here's an example code snippet that demonstrates how to authenticate and list all your documents using the Dynalist API.
const apikey = 'YOUR_API_KEY_HERE';
// Authenticate using the API key
const headers = new Headers({'Content-Type': 'application/json', 'Authorization': `Token ${apikey}`});
// Fetch all documents
fetch('https://dynalist.io/api/v1/file/list', {headers}).then(response => {
return response.json();
}).then(data => {
console.log(data);
});
Examples
Here are some other examples of API endpoints that you can use with the Dynalist API:
Create a new document
const apikey = 'YOUR_API_KEY_HERE';
const name = 'My New Document';
// Authenticate using the API key
const headers = new Headers({'Content-Type': 'application/json', 'Authorization': `Token ${apikey}`});
// Create the new document
fetch('https://dynalist.io/api/v1/file/create', {method: 'POST', headers, body: JSON.stringify({title: name})}).then(response => {
return response.json();
}).then(data => {
console.log(data);
});
Create a new item
const apikey = 'YOUR_API_KEY_HERE';
const document_id = 'DOCUMENT_ID_HERE';
const content = 'My New Item';
const parent_id = null; // set this to null to create a top-level item
// Authenticate using the API key
const headers = new Headers({'Content-Type': 'application/json', 'Authorization': `Token ${apikey}`});
// Create the new item
fetch('https://dynalist.io/api/v1/doc/edit', {method: 'POST', headers, body: JSON.stringify({file_id: document_id, changes: [{action: 'insert', parent_id, content}]})}).then(response => {
return response.json();
}).then(data => {
console.log(data);
});
Update an item's content
const apikey = 'YOUR_API_KEY_HERE';
const document_id = 'DOCUMENT_ID_HERE';
const item_id = 'ITEM_ID_HERE';
const new_content = 'My Updated Item';
// Authenticate using the API key
const headers = new Headers({'Content-Type': 'application/json', 'Authorization': `Token ${apikey}`});
// Update the item's content
fetch('https://dynalist.io/api/v1/doc/edit', {method: 'POST', headers, body: JSON.stringify({file_id: document_id, changes: [{action: 'edit', node_id: item_id, content: new_content}]})}).then(response => {
return response.json();
}).then(data => {
console.log(data);
});
Delete an item
const apikey = 'YOUR_API_KEY_HERE';
const document_id = 'DOCUMENT_ID_HERE';
const item_id = 'ITEM_ID_HERE';
// Authenticate using the API key
const headers = new Headers({'Content-Type': 'application/json', 'Authorization': `Token ${apikey}`});
// Delete the item
fetch('https://dynalist.io/api/v1/doc/edit', {method: 'POST', headers, body: JSON.stringify({file_id: document_id, changes: [{action: 'delete', node_id: item_id}]})}).then(response => {
return response.json();
}).then(data => {
console.log(data);
});
Conclusion
The Dynalist public API provides a powerful way to programmatically interact with Dynalist, allowing developers to create custom integrations and apps that extend Dynalist's functionality. With the examples above, you should have a good starting point to explore and build on the Dynalist API in your JavaScript applications.