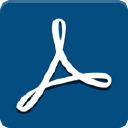
pdflayer API
Documents & ProductivityAccess PDFLayer Public API with JavaScript
PDFLayer is a great tool that allows you to integrate PDF conversion and processing functionality to your application or website. In this blog post, we will show you how to access the PDFLayer Public API using JavaScript.
Prerequisites
Before we begin, please ensure you have the following:
- A PDFLayer account
- Access to PDFLayer Public API credentials
- Basic knowledge of JavaScript
Making a Request
To make a request to the PDFLayer Public API, you need to create a new instance of the XMLHttpRequest()
object. You will also need to set the request headers and authenticate using Basic Authentication.
Here's an example code snippet to get you started:
const apiKey = "your_api_key";
const url = `https://api.pdflayer.com/api/convert?access_key=${apiKey}&document_url=https://www.website.com/sample.pdf`;
const request = new XMLHttpRequest();
request.open('GET', url, true);
request.setRequestHeader('Content-type', 'application/json');
request.setRequestHeader('Authorization', 'Basic ' + btoa(apiKey));
request.onload = function () {
const data = JSON.parse(this.response);
if (request.status == 200) {
console.log(data);
} else {
console.log('Error:', data.error.info);
}
};
request.send();
In the above example, we set the apiKey
variable to our PDFLayer Public API access key, which we will use to authenticate our request. We set the URL and then make a GET request to the API endpoint. Finally, we parse the JSON response, log the response data if the request was successful, and log an error message if the request failed.
Converting Files
PDFLayer allows you to convert various file formats to PDF. Using the Public API, you can convert files by providing the file URL or file contents as part of the request.
Let's take a look at an example of converting a HTML file to a PDF using the POST
method of PDFLayer's Conversion API.
const apiKey = "your_api_key";
const url = `https://api.pdflayer.com/api/convert`;
const request = new XMLHttpRequest();
request.open('POST', url, true);
request.setRequestHeader('Content-type', 'application/json');
request.setRequestHeader('Authorization', 'Basic ' + btoa(apiKey));
request.responseType = 'arraybuffer';
const html = "<html><body><h1>Hello world!</h1></body></html>";
const payload = JSON.stringify({
document_html: encodeURIComponent(html),
page_size: "Letter"
});
request.onload = function () {
const data = this.response;
if (request.status == 200) {
const blob = new Blob([data], {type: 'application/pdf'});
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'document.pdf';
a.click();
window.URL.revokeObjectURL(url);
} else {
console.log('Error:', data.error.info);
}
};
request.send(payload);
In the above example, we set the apiKey
variable to our PDFLayer Public API access key, which we will use to authenticate our request. We set the URL and then make a POST request to the API endpoint, including the HTML content in the payload.
We also set the responseType
to arraybuffer
since the API will return a PDF as a binary data stream. By parsing the response as an arraybuffer
, we can store it as a Blob
object that can then be downloaded using a standard a
element.
Conclusion
In this blog post, we've looked at how to access the PDFLayer Public API using JavaScript. We've covered making a request, authenticating using Basic Authentication, and converting files. With this knowledge, you can now integrate PDFLayer's powerful PDF conversion and processing functionality into your application or website. Happy coding!