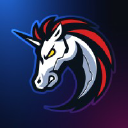
1inch
CryptocurrencyThe API for querying decentralized exchanges is a powerful tool that allows developers to integrate seamless and efficient trading functionalities into their applications. By leveraging this API, users can access a wide array of decentralized trading platforms, enabling them to retrieve market data, execute trades, and manage liquidity dynamically. It is designed to enhance user experience by providing swift access to comprehensive trading information, which is crucial in the fast-paced world of cryptocurrencies. Developers can easily incorporate this API into their projects, thereby empowering users to take advantage of decentralized finance without the need to navigate through multiple exchanges manually.
The benefits of using this API for querying decentralized exchanges include:
- Access to multiple liquidity sources, ensuring the best trade execution prices.
- Real-time market data delivery for more informed decision-making.
- Simplified integration with existing applications, reducing development time.
- Support for various tokens and trading pairs, broadening trading options.
- Enhanced security measures typical of decentralized protocols, reducing the risk of central points of failure.
Here's a JavaScript code example for calling the API:
const axios = require('axios');
const apiUrl = 'https://1inch.io/api/v4.0/1/quote'; // Replace '1' with the desired network ID
const fromToken = 'ETH'; // Token to trade from
const toToken = 'USDT'; // Token to trade to
const amount = 1000000000000000000; // Amount in wei
async function getQuote() {
try {
const response = await axios.get(apiUrl, {
params: {
fromTokenAddress: fromToken,
toTokenAddress: toToken,
amount: amount,
},
});
console.log('Quote Response:', response.data);
} catch (error) {
console.error('Error fetching quote:', error);
}
}
getQuote();