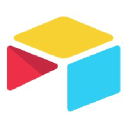
Airtable
Documents & ProductivityThe Airtable API enables developers to integrate the powerful features of Airtable into their applications, allowing for seamless data manipulation and retrieval from the popular no-code database platform. With this API, users can automate workflows, connect Airtable with other tools, and enhance collaborative efforts through real-time data synchronization. By using the Airtable API, developers can easily access tables, create new records, update existing ones, and query specific data sets, making it an essential tool for businesses looking to streamline operations and improve functionality.
Integrating with Airtable provides numerous advantages, including increased productivity, simplified data management, enhanced collaboration, and the ability to leverage Airtable’s unique capabilities across various applications. Users can benefit from a user-friendly interface alongside robust API functionalities that accommodate complex data structures and relationships. Below are some key benefits of using the Airtable API:
- Automate repetitive tasks to save time and reduce errors.
- Access a flexible and dynamic database with rich data types.
- Seamlessly integrate with other platforms for improved workflows.
- Utilize real-time updates to keep all users on the same page.
- Scale applications easily with Airtable’s intuitive design.
Here is a JavaScript code example demonstrating how to call the Airtable API to retrieve records from a specific table:
const axios = require('axios');
const baseId = 'your_base_id';
const tableName = 'your_table_name';
const apiKey = 'your_api_key';
async function fetchRecords() {
try {
const response = await axios.get(`https://api.airtable.com/v0/${baseId}/${tableName}`, {
headers: {
Authorization: `Bearer ${apiKey}`
}
});
console.log(response.data.records);
} catch (error) {
console.error('Error fetching records:', error);
}
}
fetchRecords();