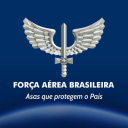
AIS Web
TransportationUsing the AISWeb API with JavaScript
The AISWeb API provides access to a range of aviation-related information and services, including weather data, flight plans, NOTAMs (Notices to Airmen), and more. In this blog post, we'll show you how to use the API with JavaScript. To get started, you'll need to sign up for an API key from the AISWeb website.
Retrieving weather data
To retrieve weather data for a particular location, you'll need to make an HTTP GET request to the weather
endpoint. Here's an example using the fetch()
method:
const apiKey = 'YOUR_API_KEY';
const location = 'SBGR'; // airport code for São Paulo/Guarulhos International Airport
fetch(`http://www.aisweb.aer.mil.br/api/api/weather?api_key=${apiKey}&location=${location}`)
.then(response => response.json())
.then(data => {
console.log(data);
});
This will retrieve the current weather data for São Paulo/Guarulhos International Airport and log it to the console.
Retrieving NOTAMs
To retrieve NOTAMs for a particular airport or area, you'll need to make an HTTP GET request to the notam
endpoint. Here's an example:
const apiKey = 'YOUR_API_KEY';
const location = 'SBSP'; // airport code for São Paulo Congonhas Airport
fetch(`http://www.aisweb.aer.mil.br/api/api/notam?api_key=${apiKey}&location=${location}`)
.then(response => response.json())
.then(data => {
console.log(data);
});
This will retrieve the current NOTAMs for São Paulo Congonhas Airport and log them to the console.
Retrieving aeronautical charts
To retrieve aeronautical charts for a particular airport or area, you'll need to make an HTTP GET request to the chart
endpoint. Here's an example:
const apiKey = 'YOUR_API_KEY';
const location = 'SBGR'; // airport code for São Paulo/Guarulhos International Airport
const chartType = 'WAC'; // chart type - possible values: WAC, TPC
fetch(`http://www.aisweb.aer.mil.br/api/api/chart?api_key=${apiKey}&location=${location}&chart_type=${chartType}`)
.then(response => {
const fileUrl = response.headers.get('Content-Disposition').match(/filename=(.*)/)[1];
return response.blob().then(blob => ({ fileUrl, blob }));
})
.then(({ fileUrl, blob }) => {
const objectUrl = window.URL.createObjectURL(blob);
window.open(objectUrl, '_blank');
})
This will retrieve the World Aeronautical Chart (WAC) for São Paulo/Guarulhos International Airport and open it in a new tab.
Conclusion
Using the AISWeb API with JavaScript can provide valuable aviation-related information and services. By following the example code above, you can get started with making API calls and accessing the data you need.