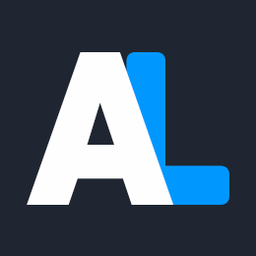
AniList
AnimeExploring the AniList API with JavaScript
If you are an anime or manga fan, then you might have heard of the AniList website. AniList provides a comprehensive database of anime and manga information, recommendations, and user reviews. But did you know that AniList also provides a public API that allows you to access its data programmatically?
In this blog post, we will explore the AniList API and how to use it with JavaScript. We will cover the basics of the API, authentication, and provide examples of how to make API requests.
Basics of the AniList API
The AniList API is a RESTful API that provides JSON data. You can use the API to access anime, manga, characters, staff, studios, and more. The API documentation is available at https://anilist.gitbook.io/anilist-apiv2-docs/. Make sure to read the documentation carefully before making any API requests.
Authentication
Before making any requests, you need to authenticate with the API. To authenticate, you need to obtain an access token by following the instructions provided in the documentation. Once you have an access token, you can include it in your API requests using the Authorization header:
const axios = require('axios');
const accessToken = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX';
const config = {
headers: { 'Authorization': `Bearer ${accessToken}` }
};
axios.get('https://anilist.co/api/v2/user', config)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Example API Requests
Here are some examples of how to make API requests using the AniList API with JavaScript:
Search Anime
To search for anime by title, you can use the following code:
axios.get('https://anilist.co/api/v2/anime/search', {
params: {
'query': 'Attack on Titan'
},
headers: {'Authorization': `Bearer ${accessToken}`}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Get Anime Information
To get information about a specific anime, you can use the following code:
axios.get('https://anilist.co/api/v2/anime/16498', {
headers: {'Authorization': `Bearer ${accessToken}`}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Get Anime Recommendations
To get anime recommendations based on a specific anime, you can use the following code:
axios.get('https://anilist.co/api/v2/anime/16498/recommendations', {
headers: {'Authorization': `Bearer ${accessToken}`}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Get User Information
To get information about a specific AniList user, you can use the following code:
axios.get('https://anilist.co/api/v2/user?name=UserName', {
headers: {'Authorization': `Bearer ${accessToken}`}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Update User List
To update a user's anime or manga list, you can use the following code:
axios.post('https://anilist.co/api/v2/user/list/update', {
'anime': [
{
'id': 16498,
'list_status': 'completed'
}
]
}, {
headers: {'Authorization': `Bearer ${accessToken}`}
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
Conclusion
The AniList API provides a powerful way to access anime and manga data programmatically. With JavaScript, you can easily make API requests and retrieve data to create your own anime or manga app or website. Make sure to read the API documentation carefully and follow best practices when making API requests. Happy coding!