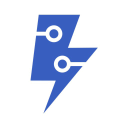
π Documentation & Examples
Everything you need to integrate with ApiFlash
π Quick Start Examples
// ApiFlash API Example
const response = await fetch('https://apiflash.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
How to use the Apiflash API
Apiflash is a public API that allows you to easily take screenshots of any website. This can be particularly useful if you're building a website that needs to display previews of other sites, or if you need to generate screenshots for a blog post or article.
Here's a quick guide on how to use the Apiflash API with JavaScript, along with some example code to help you get started.
Step 1: Sign up for an API key
Before you can start using the Apiflash API, you'll need to sign up for an API key. You can do this by visiting the Apiflash website and clicking the "Get an API Key" button. Once you've signed up, you'll be able to access your API key through your account dashboard.
Step 2: Use the API endpoint to create a screenshot
Once you have your API key, you can use it to create a screenshot of any website. To do this, you'll need to make a GET request to the API endpoint, providing the URL of the website you want to screenshot, along with your API key.
Here's an example of how you can use the API endpoint with JavaScript using the fetch()
function:
const apiUrl = `https://api.apiflash.com/v1/urltoimage?access_key=${YOUR_API_KEY}&url=https://example.com`;
fetch(apiUrl)
.then(response => response.blob())
.then(blob => {
const img = document.createElement('img');
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
});
In the example above, we're using the fetch()
function to make a GET request to the Apiflash API endpoint. We're passing in the URL of the website we want to screenshot, along with our API key. Once we get a response back from the API, we're using the blob()
function to convert the response into a blob object, and then creating a new <img>
element and appending it to the page.
Step 3: Customize the screenshot
The Apiflash API allows you to customize the screenshot in a number of ways, such as by specifying the viewport size, device type, and more. Here's an example of how you can customize the screenshot using some of the available parameters:
const apiUrl = `https://api.apiflash.com/v1/urltoimage?access_key=${YOUR_API_KEY}&url=https://example.com&full_page=false&width=1200&height=800&device=desktop`;
fetch(apiUrl)
.then(response => response.blob())
.then(blob => {
const img = document.createElement('img');
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
});
In this example, we're specifying the full_page
parameter to false
, which means that we'll only capture the visible part of the website. We're also setting the width
and height
parameters to 1200 and 800 respectively, and using the device
parameter to specify that we want to capture the desktop view of the website.
Conclusion
That's it! Using the Apiflash API with JavaScript is a simple and effective way to take screenshots of any website. Whether you're building a web app or just need to generate some screenshots for a blog post, this API is a great tool to have in your arsenal.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes