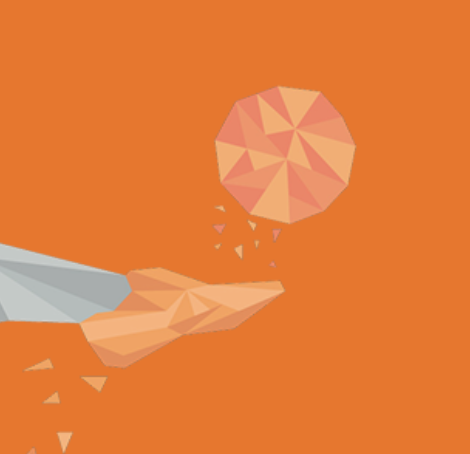
API-FOOTBALL
Sports & FitnessIntroduction to api-football.com documentation
api-football.com is a free football API that provides real-time football data, including live scores, game statistics, and historical data. Their API supports many different sports, including football, basketball, and hockey. In this article, we will explore some of their public API documentation and provide examples of how to use their APIs in JavaScript.
Getting Started
To start using the api-football.com API, you will need to sign up for a free account on their website. Once you have signed up and created an API key, you can start exploring their API documentation.
The documentation includes a list of available endpoints, along with their parameters, response formats, and authentication methods. You can use these endpoints to access various data points related to football, including real-time scores, fixtures, team information, player stats, and more.
Example API calls in JavaScript
In this section, we will provide some examples of how to use the api-football.com API in JavaScript. These examples assume that you are using the axios library to make HTTP requests.
Get Real-Time Scores
To get real-time football scores, you can use the /v2/fixtures/live
endpoint. This endpoint returns a list of fixtures that are currently in play.
const axios = require('axios');
const apiUrl = 'https://api-football-v1.p.rapidapi.com/v2/fixtures/live';
const apiOptions = {
headers: {
'x-rapidapi-host': 'api-football-v1.p.rapidapi.com',
'x-rapidapi-key': 'your-api-key',
},
params: {
timezone: 'Europe/London',
league: '39',
},
};
axios
.get(apiUrl, apiOptions)
.then(response => {
console.log(response.data.api.fixtures);
})
.catch(err => {
console.log(err);
});
Get Fixture Information
To get information about a specific fixture, you can use the /v2/fixtures/{fixture_id}
endpoint. This endpoint returns detailed information about a specific fixture, including team lineups, goals, cards, and substitutions.
const axios = require('axios');
const fixtureId = '157525';
const apiUrl = `https://api-football-v1.p.rapidapi.com/v2/fixtures/${fixtureId}`;
const apiOptions = {
headers: {
'x-rapidapi-host': 'api-football-v1.p.rapidapi.com',
'x-rapidapi-key': 'your-api-key',
},
};
axios
.get(apiUrl, apiOptions)
.then(response => {
console.log(response.data.api.fixtures);
})
.catch(err => {
console.log(err);
});
Get Standings for a League
To get league standings, you can use the /v2/leagueTable/{league_id}
endpoint. This endpoint returns a table of standings for a specific league, including points, played games, goals, and more.
const axios = require('axios');
const leagueId = '39';
const apiUrl = `https://api-football-v1.p.rapidapi.com/v2/leagueTable/${leagueId}`;
const apiOptions = {
headers: {
'x-rapidapi-host': 'api-football-v1.p.rapidapi.com',
'x-rapidapi-key': 'your-api-key',
},
};
axios
.get(apiUrl, apiOptions)
.then(response => {
console.log(response.data.api.standings);
})
.catch(err => {
console.log(err);
});
Conclusion
In this article, we have explored some of the public API documentation available on api-football.com. We have provided examples of how to use their APIs in JavaScript, including how to get real-time scores, fixture information, and league standings.
api-football.com provides a powerful and flexible API that can be used to access a wide range of football-related data. With the help of their documentation and some simple JavaScript code, you can start building your own football app or website today.