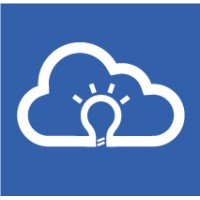
Apptigent PowerTools
DevelopmentExploring Public APIs with AppTigent
Are you tired of manually collecting and organizing data? AppTigent offers a suite of powerful APIs that provide easy access to data and automation capabilities. In this blog post, we'll explore the features and functionality of AppTigent's public API documentation for PowerTools.
Getting Started
To get started, we need to register and get an API key from AppTigent. Once we have an API key, we can use any of their APIs. Let's use the PowerTools API, which provides a set of tools for web development and automation.
To use the PowerTools API, we can simply make a GET request to their endpoint. For example, we can retrieve the weather forecast for a particular location:
const endpoint = 'https://api.apptigent.com/powertools/weather';
const apiKey = 'YOUR_API_KEY';
const location = 'New York, NY USA';
fetch(`${endpoint}?apiKey=${apiKey}&location=${location}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above example, we send a GET request to the PowerTools weather endpoint, passing our API key and the desired location as query parameters. We then convert the resulting JSON response to an object and log it to the console.
API Features
The PowerTools API includes a wide range of tools for web development and automation, including:
- Image manipulation: resize, crop, and transform images
- PDF manipulation: merge, split, and transform PDFs
- Video manipulation: transcode, convert, and edit videos
- Text extraction: extract text from PDFs, images, and videos
- OCR: extract text from scanned documents
- Web scraping: extract data from websites
- and many more
Example API Calls
Let's take a look at some additional example API calls. We can use the image manipulation API to resize an image:
const endpoint = 'https://api.apptigent.com/powertools/image/resize';
const apiKey = 'YOUR_API_KEY';
const imageUrl = 'https://example.com/image.png';
const width = 500;
const height = 500;
fetch(`${endpoint}?apiKey=${apiKey}&imageUrl=${imageUrl}&width=${width}&height=${height}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above example, we send a GET request to the PowerTools image resize endpoint, passing our API key, the image URL, and the desired width and height as query parameters.
We can also use the Web Scraper API to extract data from a website:
const endpoint = 'https://api.apptigent.com/powertools/webscraper';
const apiKey = 'YOUR_API_KEY';
const url = 'https://example.com';
const selector = 'h1';
fetch(`${endpoint}?apiKey=${apiKey}&url=${url}&selector=${selector}`)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error(error);
});
In the above example, we send a GET request to the PowerTools web scraper endpoint, passing our API key, the website URL, and the CSS selector for the data we want to extract.
Conclusion
AppTigent's PowerTools API provides a powerful set of tools for web development and automation. With a few lines of code, we can easily access data and automate tedious tasks. Give it a try today and see how it can streamline your workflow!