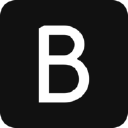
Base API
DevelopmentUsing the Base API
The Base API makes it easy to power your application with customer data from a variety of different sources. In this tutorial, we'll go over how to use the API with JavaScript.
Getting Started
To start using the Base API, you'll need to create an account and obtain an API key. Once you have your key, you'll be able to use it to authenticate requests to the API.
Here's an example of how to authenticate with the Base API using JavaScript:
const apiKey = '<your-api-key>';
const headers = {
Authorization: `Bearer ${apiKey}`,
};
With this header set, you'll be able to make requests to the API.
Retrieving Customer Data
Let's say we want to retrieve information about a specific customer. Here's an example of how to do that using the Base API:
const customerId = '<customer-id>';
const url = `https://api.base-api.io/v1/customers/${customerId}`;
fetch(url, { headers })
.then(response => response.json())
.then(data => console.log(data));
This will retrieve customer data and log it to the console.
Updating Customer Data
If you need to update a customer record, you can do that using the PUT
method:
const customerId = '<customer-id>';
const url = `https://api.base-api.io/v1/customers/${customerId}`;
const body = {
email: 'new-email@example.com',
firstName: 'New',
lastName: 'Name',
};
fetch(url, {
headers,
method: 'PUT',
body: JSON.stringify(body),
})
.then(response => response.json())
.then(data => console.log(data));
Adding a New Customer
Finally, let's see how to add a new customer to the Base API:
const url = 'https://api.base-api.io/v1/customers';
const body = {
email: 'new-customer@example.com',
firstName: 'New',
lastName: 'Customer',
};
fetch(url, {
headers,
method: 'POST',
body: JSON.stringify(body),
})
.then(response => response.json())
.then(data => console.log(data));
This will add a new customer to the API and log the response to the console.
Conclusion
That's it! Using the Base API with JavaScript is straightforward and simple. With these examples, you'll be able to easily retrieve and update customer data in your own applications.