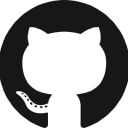
Binance
CryptocurrencyHow to Use Binance Public API with JavaScript
If you are interested in developing cryptocurrency trading applications, then Binance's official API documentation is the perfect starting point for you. In this article, we will guide you through the steps of accessing and using the Binance public API with JavaScript.
Introduction to Binance Public API
Binance is one of the world's leading cryptocurrency exchange platforms that offers its users an API that allows developers to connect to their trading database and execute trades automatically. The Binance public API consists of several endpoints that can be accessed and utilized for various purposes, including getting cryptocurrency market data and trading orders execution.
Setting up the Environment
To use Binance's public API with JavaScript, you will need to install some essential libraries such as axios, as this, along with other libraries, will enable you to make HTTP requests and handle the returned data easily. Here is an example of how to set up your JavaScript environment using Node.js:
const axios = require('axios');
Handling the API Endpoints
The Binance public API supports different endpoints that return different types of data, including market data, trade data, and account data. Here are some of the endpoints that you can use with JavaScript:
Get Ticker Price
This endpoint returns the ticker price for all trading pairs on Binance.
axios.get('https://api.binance.com/api/v3/ticker/price')
.then(response => console.log(response.data))
.catch(error => console.log(error));
Get Trades
This endpoint returns recent trades for a specified trading pair.
axios.get('https://api.binance.com/api/v3/trades', {
params: {
symbol: 'BTCUSDT',
limit: 5
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error));
Get Order Book
This endpoint returns the order book for a specified trading pair.
axios.get('https://api.binance.com/api/v3/depth', {
params: {
symbol: 'BTCUSDT',
limit: 5
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error));
Get Klines Data
This endpoint returns Klines data for a specified trading pair.
axios.get('https://api.binance.com/api/v3/klines', {
params: {
symbol: 'BTCUSDT',
interval: '1h',
limit: 5
}
})
.then(response => console.log(response.data))
.catch(error => console.log(error));
Placing an Order
This endpoint allows you to place an order for a particular trading pair.
axios.post('https://api.binance.com/api/v3/order', {
symbol: 'BTCUSDT',
side: 'BUY',
type: 'LIMIT',
timeInForce: 'GTC',
quantity: 0.001,
price: 35000
})
.then(response => console.log(response.data))
.catch(error => console.log(error));
Conclusion
In this guide, we have shown you how to get started using Binance's public API with JavaScript. We have covered some of the essential endpoints that you need to know when developing cryptocurrency trading applications using Binance. Remember to keep your API key safe and secure and use it wisely when making requests to avoid any unauthorized access. Good luck!