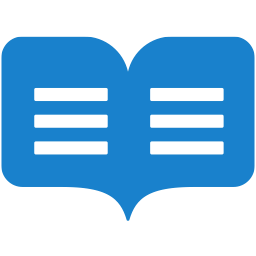
Bitfinex
CryptocurrencyBitfinex API Documentation
Are you looking for a reliable cryptocurrency exchange platform with a powerful API to help you access trading data? Look no further than Bitfinex!
Introduction
Bitfinex provides a public REST API allowing users to retrieve information about currency pairs, trading values, and more. It's designed to provide a fast, reliable way to interact with Bitfinex's trading engine.
Getting Started
First, you'll need to create an account with Bitfinex. Once you've done that, you can access the API using the endpoint URL, https://api.bitfinex.com/v1/. You can also refer to our official API documentation at https://docs.bitfinex.com/docs/introduction for more information.
Example API Calls in JavaScript
Public API Calls
Get Ticker
const url = "https://api.bitfinex.com/v1/pubticker/btcusd";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Get Trades
const url = "https://api.bitfinex.com/v1/trades/btcusd?limit_trades=2";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Get Order Book
const url = "https://api.bitfinex.com/v1/book/btcusd";
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Authenticated API Calls
You will need to create an API secret key in order to make authenticated API calls. Here's an example of how to do that:
const crypto = require("crypto");
const apiKey = "[YOUR_API_KEY]";
const apiSecret = "[YOUR_API_SECRET]";
const getNonce = () => Math.round(new Date().getTime() / 1000);
const createSignature = (nonce) => {
const rawSignature = `AUTH${nonce}`;
return crypto.createHmac("sha384", apiSecret).update(rawSignature).digest("hex");
};
const getHeaders = (url) => {
const nonce = getNonce();
const signature = createSignature(nonce);
return {
"X-BFX-APIKEY": apiKey,
"X-BFX-PAYLOAD": new Buffer('{"request":"/v1' + url + '","nonce":"' + nonce + '"}').toString('base64'),
"X-BFX-SIGNATURE": signature,
};
};
Get Account Balance
const url = "/balances";
const headers = getHeaders(url);
fetch(`https://api.bitfinex.com/v1${url}`, { headers })
.then(response => response.json())
.then(data => console.log(data));
Place an Order
const url = "/order/new";
const symbol = "btcusd";
const amount = 1;
const price = 60000;
const side = "buy";
const type = "exchange limit";
const payload = JSON.stringify({
symbol,
amount,
price,
side,
type,
});
const headers = getHeaders(url);
fetch(`https://api.bitfinex.com/v1${url}`, { body: payload, headers, method: "POST" })
.then(response => response.json())
.then(data => console.log(data));
Conclusion
Bitfinex provides a robust and user-friendly API for accessing exchange data and executing trades programmatically. By following the examples above, you'll be able to quickly and easily interact with Bitfinex's API in JavaScript. Happy trading!