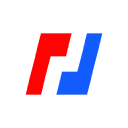
Bitmex
CryptocurrencyExploring BitMEX Public API with JavaScript
BitMEX is a leading cryptocurrency exchange that provides trading services for Bitcoin and other digital assets. The platform offers a powerful API that can be used to build custom trading bots, integrate third-party applications, and create advanced trading strategies.
In this article, we'll explore BitMEX's public API and walk you through some JavaScript code examples to help you get started.
Prerequisites
Before we dive into the code, you'll need to ensure that you have the following prerequisites installed:
- Node.js (v8 or later)
- npm (Node package manager)
Getting Started
To start using BitMEX's public API, you'll need to obtain an API key from the BitMEX website. Once you have your API key, you can start making requests to the various API endpoints.
To make requests to the BitMEX API, we'll be using the request
module, which is a popular HTTP client library for Node.js. Here's how to install it:
$ npm install request
Examples
1. Get the BitMEX order book
The following code example demonstrates how to retrieve the order book for the XBT/USD trading pair:
const request = require('request');
const baseUrl = 'https://www.bitmex.com/api/v1';
const symbol = 'XBTUSD';
request(`${baseUrl}/orderBook/L2?symbol=${symbol}&depth=10`, (error, response, body) => {
if (error) {
console.error(error);
} else {
console.log(JSON.parse(body));
}
});
2. Get the BitMEX recent trades
This code example shows you how to retrieve the recent trades for the XBT/USD trading pair:
const request = require('request');
const baseUrl = 'https://www.bitmex.com/api/v1';
const symbol = 'XBTUSD';
request(`${baseUrl}/trade?symbol=${symbol}&count=10`, (error, response, body) => {
if (error) {
console.error(error);
} else {
console.log(JSON.parse(body));
}
});
3. Get the BitMEX ticker data
This code example demonstrates how to retrieve the current ticker data for the XBT/USD trading pair:
const request = require('request');
const baseUrl = 'https://www.bitmex.com/api/v1';
const symbol = 'XBTUSD';
request(`${baseUrl}/instrument?symbol=${symbol}&columns=lastPrice`, (error, response, body) => {
if (error) {
console.error(error);
} else {
console.log(JSON.parse(body));
}
});
4. Get the BitMEX historical data
This code example demonstrates how to retrieve historical data for the XBT/USD trading pair:
const request = require('request');
const baseUrl = 'https://www.bitmex.com/api/v1';
const symbol = 'XBTUSD';
const startTime = new Date('2022-01-01T00:00:00.000Z').toISOString();
const endTime = new Date('2022-01-05T00:00:00.000Z').toISOString();
request(`${baseUrl}/trade/bucketed?binSize=1d&partial=false&symbol=${symbol}&count=100&reverse=true&startTime=${startTime}&endTime=${endTime}`, (error, response, body) => {
if (error) {
console.error(error);
} else {
console.log(JSON.parse(body));
}
});
Conclusion
In this article, we've shown you how you can use JavaScript to interact with the BitMEX public API. With these examples, you can get started building your trading bot or integrating BitMEX data into your own applications.
Remember to always exercise caution and take appropriate risk management measures when building and using trading bots. Good luck, and happy coding!