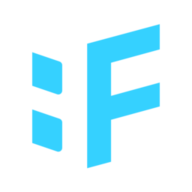
BlockFacts API
CryptocurrencyUsing Blockfacts.io Public API - An Overview
Blockfacts.io offers a comprehensive set of APIs that provide real-time and historical cryptocurrency data. You can use these APIs to get cryptocurrency prices, exchange rates, historical data, order book data, and more. In this post, we will explore how to use the Blockfacts.io public API with JavaScript.
Getting Started
Before we dive into the API examples, we need to obtain an API key from Blockfacts.io. To get an API key, sign up for a free account on the Blockfacts.io website, and then navigate to the API section to create a new API key.
API Endpoints
Blockfacts.io offers several API endpoints that provide different types of data. Here we list a few essential endpoints to get started:
/v0/rates
: Returns the current exchange rates for the requested currency pairs/v0/tickers
: Returns the latest ticker data for all supported cryptocurrencies and fiat pairs/v0/markets
: Returns the list of all supported markets and their details/v0/history
: Returns historical OHLCV data for a requested market and a given time range with optional filters and intervals.
API Examples
Here we will provide a few examples of how to use the Blockfacts.io public API with JavaScript. For these examples, we will be using the axios
library to make HTTP requests.
Example 1: Getting Current Exchange Rates
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const CURRENCY_PAIRS = ['BTC-USD', 'ETH-USD'];
const URL = `https://api.blockfacts.io/v0/rates?apikey=${API_KEY}&pairs=${CURRENCY_PAIRS.join(',')}`;
axios.get(URL)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
In this example, we are getting the current exchange rates for two currency pairs: BTC-USD and ETH-USD. We are passing our API key as a query parameter and making a GET request to the /v0/rates
endpoint. The response from the API will contain the exchange rates for the requested currency pairs.
Example 2: Getting Latest Ticker Data
const axios = require('axios');
const API_KEY = 'YOUR_API_KEY';
const URL = `https://api.blockfacts.io/v0/tickers?apikey=${API_KEY}`;
axios.get(URL)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
In this example, we are getting the latest ticker data for all supported cryptocurrencies and fiat pairs. We are passing our API key as a query parameter and making a GET request to the /v0/tickers
endpoint. The response from the API will contain the latest ticker data for all supported pairs.
Example 3: Getting Historical OHLCV Data
const axios = require('axios');
const moment = require('moment');
const API_KEY = 'YOUR_API_KEY';
const MARKET = 'BINANCE-ETH-BTC';
const FROM = moment().subtract(7, 'days').unix();
const TO = moment().unix();
const URL = `https://api.blockfacts.io/v0/history?apikey=${API_KEY}&market=${MARKET}&from=${FROM}&to=${TO}&interval=1d`;
axios.get(URL)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
In this example, we are getting the historical OHLCV data for the Binance ETH-BTC market for the past seven days. We are passing our API key, the market name, the time range, and the interval as query parameters and making a GET request to the /v0/history
endpoint. The response from the API will contain the OHLCV data for the requested market in the requested time range and interval.
Conclusion
Blockfacts.io provides a comprehensive set of APIs to access real-time and historical cryptocurrency data. In this post, we explored a few essential API endpoints and demonstrated how to use them with JavaScript. You can use these examples as the basis for building your own applications that leverage the power of the Blockfacts.io public API.