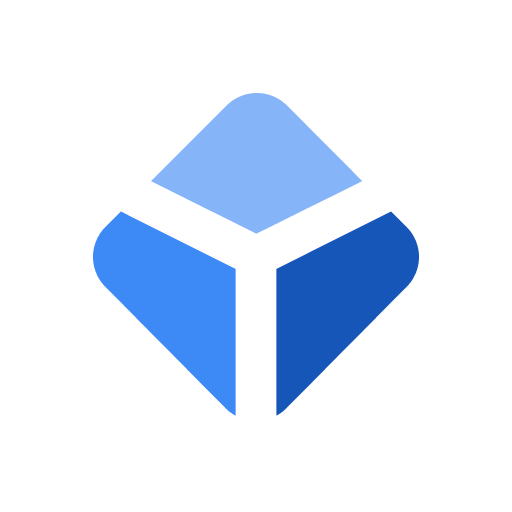
Blockchain API
CryptocurrencySend and receive payment from Blockchain Wallets, transactions, and blocks data. Explore the REST API documentation for detailed examples of the various functionality offered, such as receiving real-time market data, requesting balance information, and performing trades.
📚 Documentation & Examples
Everything you need to integrate with Blockchain API
🚀 Quick Start Examples
// Blockchain API API Example
const response = await fetch('https://www.blockchain.com/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Blockchain.com API with JavaScript
The Blockchain.com API provides developers with a set of endpoints that allow them to access real-time and historical data on cryptocurrencies, exchange rates, and other blockchain-related information. In this tutorial, we will explore the different endpoints available in this API and look at some example code written in JavaScript to help you get started.
Getting Started
To use the Blockchain.com API, you will need to sign up for an API key by creating an account on the website. Once you have an API key, you can start making requests to the API endpoints.
API Endpoints
The following are some of the most commonly used endpoints in the Blockchain.com API:
-
Bitcoin Price Index: Returns the current price of Bitcoin in a given currency. This endpoint can be used to get the current exchange rate for Bitcoin.
-
Exchange Rates: Returns the current exchange rates for multiple cryptocurrencies and fiat currencies.
-
Blocks: Returns information about individual blocks on the Bitcoin blockchain, including the hash, height, and number of transactions.
-
Transactions: Returns information about individual Bitcoin transactions, including the transaction hash, block hash, and total transaction value.
-
Wallets: Returns information about Bitcoin wallets, including the balance, number of transactions, and transaction fees.
Example Code in JavaScript
The following is an example code written in JavaScript to call the Blockchain.com API and retrieve the current Bitcoin price:
const apiKey = 'Your_API_Key';
const currency = 'USD';
fetch(`https://api.blockchain.com/v3/exchange/tickers/BTC-${currency}?apikey=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log(`1 BTC = ${data.last_trade_price} ${currency}`);
})
.catch(error => {
console.error(error);
});
In this example, we first define our API key and the currency we want to get the Bitcoin price in. We then use the fetch()
method to make a GET request to the exchange/tickers
endpoint with the Bitcoin ticker and the specified currency. We then parse the response using the response.json()
method and log the current Bitcoin price to the console.
Conclusion
In this tutorial, we explored the different endpoints available in the Blockchain.com API and saw some example code written in JavaScript to get started with the API. The Blockchain.com API provides developers with a wealth of information on the blockchain and cryptocurrencies that can be used to build a variety of applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes