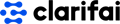
đ Documentation & Examples
Everything you need to integrate with Clarifai
đ Quick Start Examples
// Clarifai API Example
const response = await fetch('https://docs.clarifai.com/api-guide/api-overview', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Computer Vision API, brought to you by Clarifai, is a powerful tool that leverages deep learning technology to analyze and interpret the content of an image or video. So, whether your application requires image recognition, object detection or segmentation, or whether it needs to extract textual content from images, Clarifai's cloud-based Computer Vision API offers vanguard solutions endowing your applications with the ability to see and understand the world. Its user-friendly nature complemented with simplified integration features make it a highly sought-after tool in the market for developers who aim to incorporate AI-powered image/video recognition capabilities into their applications.
Expediting the automation process, the API boasts its capability to 'learn' from the data inputs and better its performance with each cycle, making its identification and interpretation skills immensely precise over time. Adhering to the highest degrees of security standards, the Computer Vision API ensures your data remains protected at all times. It is developed with a keen focus on scalability, able to handle growing business needs with constant availability and consistent response times by maintaining high-level performance even during peak-demand periods.
Benefits of using Computer Vision API:
- Simplified integration process.
- AI-powered image/video recognition capabilities.
- Self-learning model to optimize performance.
- Strict data security standards.
- Scalability to handle growing business needs.
JavaScript Code to call the API:
var request = require('request');
var options = {
url: 'https://api.clarifai.com/v2/models/aaa03c23b3724a16a56b629203edc62c/outputs',
method: 'POST',
headers: {
'Authorization': 'Key {YOUR_API_KEY}',
'Content-Type': 'application/json'
},
body: {
'inputs': [
{
'data': {
'image': {
'url': 'https://samples.clarifai.com/metro-north.jpg'
}
}
}
]
},
json: true
};
request(options, function(error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body);
}
});
Replace {YOUR_API_KEY}
with your actual API key.
Security Assessment
đ 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes