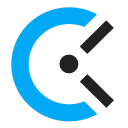
Clockify
Documents & ProductivityClockify's REST-based API is designed to facilitate seamless data integration between your applications and Clockify's powerful time tracking features. By utilizing this API, developers can effortlessly push and pull data, allowing for real-time updates and synchronization with other systems. Whether you're looking to extract time entries, manage projects, or access user reports, the API provides comprehensive endpoints to meet your requirements. This functionality not only enhances operational efficiency but also enables businesses to combine their data sources, leading to more informed decision-making.
Integrating Clockify’s API into your workflow can yield significant benefits. Some key advantages include:
- Simplified integration with existing systems and applications.
- Enhanced data accuracy through real-time updates and synchronization.
- Improved productivity by automating time tracking and reporting processes.
- Accessible and flexible data manipulation capabilities tailored to your needs.
- Comprehensive documentation and support to guide developers through implementation.
Here’s a simple JavaScript code example for calling the Clockify API to retrieve a list of workspaces:
const fetch = require('node-fetch');
const apiKey = 'YOUR_API_KEY';
const url = 'https://api.clockify.me/api/v1/workspaces';
async function getWorkspaces() {
try {
const response = await fetch(url, {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'X-Api-Key': apiKey
}
});
const workspaces = await response.json();
console.log(workspaces);
} catch (error) {
console.error('Error fetching workspaces:', error);
}
}
getWorkspaces();