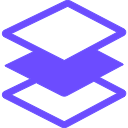
CoinAPI
CryptocurrencyAn overview of the CoinAPI Public API
CoinAPI provides a wide range of data concerning cryptocurrencies through its public API. The API can be used to retrieve real-time and historical data of different cryptocurrencies, including market data, exchange rates, and more.
To access the CoinAPI Public API, users need to have an API key. The key can be obtained by creating an account on the official CoinAPI website. Once you have your API key, you can start using the API to retrieve data.
Getting started with the CoinAPI Public API
In order to use the API, a programming language like JavaScript can be used to send requests to the CoinAPI server. The requests can be RESTful URL requests with query parameters that specify what data is requested.
Retrieving a list of all available exchanges
const fetch = require("node-fetch");
const apiKey = "API_KEY_HERE";
const endpoint = "https://rest.coinapi.io/v1/exchanges";
fetch(endpoint, {
headers: {
"X-CoinAPI-Key": apiKey
}
})
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.log(error);
});
In this example, we're requesting a list of all available exchanges supported by the CoinAPI Public API. The fetch
function sends a GET request to the specified API endpoint with the X-CoinAPI-Key
header that holds the user's API key.
Retrieving the current price of Bitcoin on a specific exchange
const fetch = require("node-fetch");
const apiKey = "API_KEY_HERE";
const endpoint = "https://rest.coinapi.io/v1/exchangerate/BTC/USD";
fetch(endpoint, {
headers: {
"X-CoinAPI-Key": apiKey
}
})
.then(response => response.json())
.then(data => {
console.log(data.rate);
})
.catch(error => {
console.log(error);
});
This code snippet shows how to retrieve the current exchange rate of Bitcoin to US Dollars on a specific exchange. In this example, the code fetches the data for the "BTC/USD" currency pair by sending a GET request to the specified API endpoint.
Conclusion
The CoinAPI Public API is a powerful tool for retrieving real-time and historical data on different cryptocurrencies and exchanges. With a good understanding of how to use the API, developers can build applications and services that rely on such data in their design. Give it a try today!