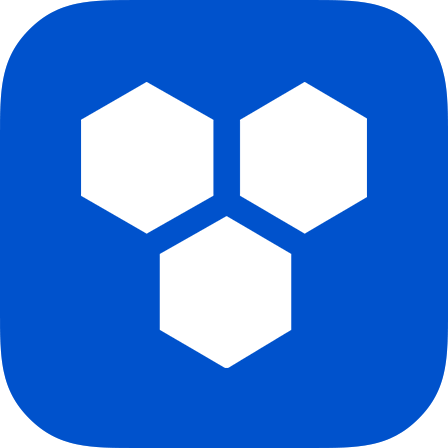
CoinLayer
CryptocurrencyIntroduction to Coinlayer API
Coinlayer is a public API that provides real-time and historical cryptocurrency exchange rates. It supports over 385 cryptocurrencies and provides data in over 25 fiat currencies. Coinlayer offers a free plan and also provides paid plans with more features.
How to use Coinlayer API
- Signup for a free account at https://coinlayer.com.
- Once you are logged in to your account, you can access the API key.
- Coinlayer API supports HTTPS requests. Use the endpoint https://api.coinlayer.com to make the requests.
API examples
Get real-time exchange rates
This example code shows how to get real-time exchange rates for Bitcoin, Ethereum, and Litecoin in USD using JavaScript.
const API_KEY = 'YOUR_API_KEY';
const CRYPTOCURRENCIES = 'BTC,ETH,LTC';
const CURRENCY = 'USD';
fetch(`https://api.coinlayer.com/live?access_key=${API_KEY}&symbols=${CRYPTOCURRENCIES}&target=${CURRENCY}`)
.then(response => response.json())
.then(data => console.log(data.rates));
Get historical exchange rates
This example code shows how to get historical exchange rates for Bitcoin, Ethereum, and Litecoin in USD for a specific date using JavaScript.
const API_KEY = 'YOUR_API_KEY';
const CRYPTOCURRENCIES = 'BTC,ETH,LTC';
const CURRENCY = 'USD';
// Date should be in YYYY-MM-DD format
const DATE = '2021-10-21';
fetch(`https://api.coinlayer.com/${DATE}?access_key=${API_KEY}&symbols=${CRYPTOCURRENCIES}&target=${CURRENCY}`)
.then(response => response.json())
.then(data => console.log(data.rates));
Convert one cryptocurrency to another
This example code shows how to convert 1 Bitcoin (BTC) to 10 Ethereum (ETH) using JavaScript.
const API_KEY = 'YOUR_API_KEY';
const FROM_CURRENCY = 'BTC';
const TO_CURRENCY = 'ETH';
const AMOUNT = 1;
fetch(`https://api.coinlayer.com/convert?access_key=${API_KEY}&from=${FROM_CURRENCY}&to=${TO_CURRENCY}&amount=${AMOUNT}`)
.then(response => response.json())
.then(data => console.log(`1 ${FROM_CURRENCY} is equal to ${data.result} ${TO_CURRENCY}`));
Conclusion
Coinlayer API makes it easy to access real-time and historical cryptocurrency exchange rates. With the help of these examples, you can easily integrate Coinlayer API with your JavaScript application.