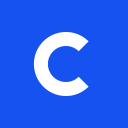
Coinbase
CryptocurrencyIntroduction to Coinbase Public API
Coinbase is a popular digital currency exchange where people can buy, sell, and store various cryptocurrencies. Coinbase provides an API that can be used by developers to access various functionalities of the exchange. The Coinbase Public API is a REST (Representational State Transfer) API that can be used to get real-time market updates, buy and sell cryptocurrencies, and manage accounts. In this article, we will explore the various components of the Coinbase Public API and show some example code in JavaScript.
Getting Started with Coinbase Public API
To get started with the Coinbase Public API, you first need to create an account on Coinbase and obtain API credentials. You can obtain API credentials by creating an API key and secret from your Coinbase account settings. Once you have obtained the credentials, you can start using the Coinbase Public API to interact with the exchange.
Example Code
Here is an example code snippet in JavaScript that shows how to obtain account information using the Coinbase Public API:
const apiKey = 'your-api-key';
const apiSecret = 'your-api-secret';
const Coinbase = require('coinbase');
const Client = Coinbase.Client;
const client = new Client({
apiKey: apiKey,
apiSecret: apiSecret
});
client.getAccount('your-account-id', function(err, account) {
console.log(account);
});
In this example, we have used the Coinbase Node.js library to interact with the Coinbase Public API. We have first created a client object by passing the API credentials to the constructor. We have then used the getAccount
method to obtain the account object, which contains information about the account balance, currency, and other details.
Conclusion
In this article, we have introduced the Coinbase Public API and shown an example of how to obtain account information using JavaScript. The Coinbase Public API provides a simple and efficient way to access various functionalities of the Coinbase exchange. By using the Coinbase Public API, developers can create applications that can buy, sell, and manage cryptocurrencies on behalf of users. If you are interested in using the Coinbase Public API, you can visit the official documentation website for more information.