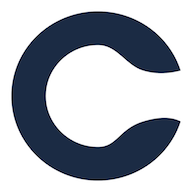
Coinlib
CryptocurrencyCoinLib API Documentation
CoinLib is a cryptocurrency information and market data provider. Using their API, developers can integrate real-time cryptocurrency market data, historical price data, and other information into their applications. In this blog post, we will explore the CoinLib API and provide code examples in JavaScript.
Base URL
The base URL for the CoinLib API is: https://coinlib.io/api/v1/
Authentication
CoinLib API does not require authentication.
Endpoints
Get List of Currencies
Returns a list of all cryptocurrencies supported by CoinLib.
const url = 'https://coinlib.io/api/v1/coinlist?key=your_api_key';
fetch(url)
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
Get Specific Cryptocurrency Data
Returns data for a specific cryptocurrency.
const url = 'https://coinlib.io/api/v1/coin?key=COIN_SYMBOL&key=your_api_key';
fetch(url)
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
Replace COIN_SYMBOL
with the symbol of the cryptocurrency you wish to retrieve data for.
Get Global Data
Returns global cryptocurrency market data.
const url = 'https://coinlib.io/api/v1/global?key=your_api_key';
fetch(url)
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
Get Historical Data
Returns historical cryptocurrency price data.
const url = 'https://coinlib.io/api/v1/coin/history?key=COIN_SYMBOL&interval=INTERVAL_IN_MINUTES&start=TIMESTAMP_IN_SECONDS&end=TIMESTAMP_IN_SECONDS&key=your_api_key';
fetch(url)
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
Replace the following variables in the URL:
COIN_SYMBOL
with the symbol of the cryptocurrency you wish to retrieve data for.INTERVAL_IN_MINUTES
with the time interval for each data point, e.g. 30 for 30-minute intervals.START_TIMESTAMP_IN_SECONDS
with the start time in seconds since the Unix epoch.END_TIMESTAMP_IN_SECONDS
with the end time in seconds since the Unix epoch.
Conclusion
CoinLib API provides a wealth of cryptocurrency market data that developers can use to create innovative applications and services. In this blog post, we have covered some of the most essential API endpoints and provided code examples in JavaScript. Happy coding!