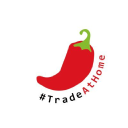
Coinpaprika
CryptocurrencyExploring Coinpaprika's Public API Documentation
Coinpaprika is a popular and reliable cryptocurrency market data provider. They offer a public API that allows developers to use their data to build a wide range of applications. In this blog, we will explore the Coinpaprika's Public API documentation and write several code snippets in JavaScript.
Getting Started
Before we can use Coinpaprika's public API, we need to register and obtain an API key. Once we have the API key, we can start using the API. Coinpaprika's API supports multiple commands which include getting ticker information, historical prices, exchanges info, and more.
JavaScript Code Snippets
Fetching Ticker Information
const apiKey = 'YOUR_API_KEY';
const url = `https://api.coinpaprika.com/v1/ticker/btc-bitcoin?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
The above code fetches the ticker information for bitcoin using the fetch
function. It also validates the response and logs it to the console. Replace YOUR_API_KEY
with the API key that you obtained from Coinpaprika.
Getting Historical Prices
const apiKey = 'YOUR_API_KEY';
const interval = '1h';
const url = `https://api.coinpaprika.com/v1/coins/btc-bitcoin/ohlcv/historical?start=2019-01-01&end=2019-12-31&interval=${interval}&api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code snippet demonstrates how to get historical prices for bitcoin using the fetch
function. Replace YOUR_API_KEY
with the API key and interval
with a different time interval if desired.
Getting Exchanges Information
const apiKey = 'YOUR_API_KEY';
const url = `https://api.coinpaprika.com/v1/exchanges?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code snippet retrieves the exchange information using the fetch
function. Replace YOUR_API_KEY
with your API key.
Getting Twitter Information
const apiKey = 'YOUR_API_KEY';
const url = `https://api.coinpaprika.com/v1/coins/btc-bitcoin/twitter?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
This code retrieves Twitter information for bitcoin using the fetch
function. Replace YOUR_API_KEY
with your API key.
Conclusion
In this blog, we explored Coinpaprika's public API documentation and wrote several code snippets in JavaScript to demonstrate how it can be used in an application. Coinpaprika's Public API is a great tool for developers who are building cryptocurrency-related applications or websites.