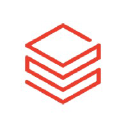
Databricks
DevelopmentThe Databricks API is an essential tool for developers and data engineers looking to streamline their workflows within their Databricks environment. This powerful service allows users to efficiently manage crucial components of their Databricks account, including clusters, notebooks, jobs, and workspaces. By utilizing this API, organizations can automate routine tasks, enhance productivity, and maintain better control over their big data processing activities. With detailed documentation available at Databricks API Documentation, users can access comprehensive guides and references to help implement and integrate the API seamlessly into their existing applications.
The benefits of leveraging the Databricks API extend beyond basic management functionalities. Key benefits include:
- Streamlined automation of cluster and job management.
- Simplified management of notebooks and collaborative projects.
- Enhanced scalability for handling large datasets and complex computations.
- Improved monitoring and logging of jobs and performance metrics.
- Seamless integration with other tools and services within your data workflow.
Here's a sample JavaScript code snippet to demonstrate how to call the Databricks API to list all clusters in your account:
const fetch = require('node-fetch');
const url = 'https://YOUR-DATABRICKS-WORKSPACE-URL/api/2.0/clusters/list';
const token = 'YOUR-DATABRICKS-ACCESS-TOKEN';
const fetchClusters = async () => {
const response = await fetch(url, {
method: 'GET',
headers: {
'Authorization': `Bearer ${token}`,
'Content-Type': 'application/json'
}
});
if (!response.ok) {
throw new Error(`Error: ${response.statusText}`);
}
const data = await response.json();
console.log(data);
};
fetchClusters().catch(error => console.error('Error fetching clusters:', error));