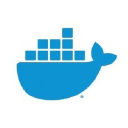
Docker Hub
DevelopmentThe Docker Hub API is a robust tool designed to easily interact with Docker Hub's functionalities and resources. Docker Hub, known as a trusted cloud-based application registry, provides an end-to-end platform that assists developers in building, sharing, securing, and running their applications with the backing of Docker's comprehensive ecosystem. By accessing the API, developers can streamline their work by programmatically managing user accounts, teams, organizations, repositories, and more directly within their coding environments. Detailed documentation is readily available on Docker's official developer site.
Leveraging the Docker Hub API offers several key benefits:
- Ease of Integration: It provides a standard interface that can be effortlessly integrated into your development process.
- Automated Management: You can automate the management of repositories, containers, and applications.
- DevOps-friendly: It supports continuous integration and continuous deployment (CI/CD) strategies.
- Increased Productivity: The API assists in simplifying complex tasks, thereby speeding up application delivery times.
- Robust Security: Docker Hub API helps in implementing security measures, including managing team access and securing the application delivery process.
To call the Docker Hub API using JavaScript, refer to the sample code snippet below:
var axios = require('axios');
var config = {
method: 'get',
url: 'https://hub.docker.com/v2/repositories/library/',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer {your_token_here}'
}
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error) {
console.error(error);
});
In the above code snippet, we're using the popular Axios library to send a GET request to the Docker Hub API. Be sure to replace {your_token_here}
with your actual Docker API Bearer token.