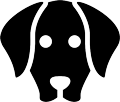
Dog API
AnimalsWorking with the Dog API
If you're a dog lover, then the Dog API is the perfect tool for you. This is an open source API that allows you to fetch various information about dogs. You can get information on breeds, sub-breeds, and random dog images among other things. In this blog post, we'll explore how to work with this API using JavaScript and show examples of various API calls.
Getting Started
To get started, you'll need to register an account on the Dog API website, https://dog.ceo/. Once you have an account, you can create an API key to use the API.
Making API Calls
The Dog API is a REST API, which means you can make HTTP requests to it to get information. You can use the fetch
API in JavaScript to make these requests. Here's an example of fetching a list of all the breeds of dogs.
fetch('https://dog.ceo/api/breeds/list/all')
.then(response => response.json())
.then(data => console.log(data));
This will log an object containing the breeds and sub-breeds of dogs to the console.
{
"message": {
"affenpinscher": [],
"african": [],
"airedale": [],
...
},
"status": "success"
}
To display these breeds on a web page, you can use JavaScript to create HTML elements dynamically. Here's an example of how to do that with a dropdown menu.
const dropdown = document.querySelector('#breeds');
fetch('https://dog.ceo/api/breeds/list/all')
.then(response => response.json())
.then(data => {
const breedsObj = data.message;
for (let breed in breedsObj) {
const option = document.createElement('option');
option.value = breed;
option.textContent = breed.charAt(0).toUpperCase() + breed.slice(1);
dropdown.appendChild(option);
}
});
This will create a dropdown menu with all the breeds of dogs in the API.
Retrieving Images
One of the most popular features of the Dog API is retrieving random images of dogs. You can do that with the https://dog.ceo/api/breeds/image/random
endpoint. Here's an example of how to retrieve and display a random dog image using JavaScript.
const img = document.querySelector('#dog-img');
fetch('https://dog.ceo/api/breeds/image/random')
.then(response => response.json())
.then(data => {
img.src = data.message;
img.alt = 'A random dog image';
});
This will retrieve a random dog image from the API and display it on the web page.
Conclusion
In this blog post, we've explored how to use the Dog API to fetch breeds of dogs and random dog images. We've shown examples of how to make API calls using JavaScript and how to display the results on a web page. The Dog API is a fun and educational tool that can be used to learn more about our canine friends.