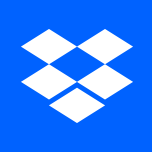
Dropbox
Cloud Storage & File SharingIntegrate your apps with the content and collaboration solution trusted by more than 500 million users and 300,000 teams. Whether you’re building your business or optimizing internal workflows, the DBX Platform lets you add Dropbox features to your apps, such as file storage, sharing, previews, and search.
📚 Documentation & Examples
Everything you need to integrate with Dropbox
🚀 Quick Start Examples
// Dropbox API Example
const response = await fetch('https://www.dropbox.com/developers', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Working with Dropbox API in JavaScript
Dropbox is a popular cloud storage platform that allows users to store and access their files from anywhere. The Dropbox API provides a way to interact with Dropbox programmatically, giving developers access to various features such as file uploads, downloads, and folder operations.
In this blog post, we'll explore how to work with the Dropbox API in JavaScript and provide examples of common use cases.
Creating a Dropbox API App
Before we get started, we need to create an app on the Dropbox App Console. Follow these steps to create your Dropbox API app:
- Go to the Dropbox App Console and click the "Create app" button.
- Choose the type of app you want to create (e.g., Scoped access or Full Dropbox access).
- Enter a name for your app and click the "Create app" button.
You will be redirected to the app configuration page where you can generate access tokens, configure app settings, and view the app's API documentation.
Authenticating with the Dropbox API
To use the Dropbox API, we first need to authenticate our app. Dropbox supports the OAuth 2.0 authentication flow, which requires users to grant permission to our app to access their files.
Here's an example of how to authenticate with the Dropbox API using JavaScript:
const CLIENT_ID = '<your-client-id>';
const REDIRECT_URI = '<your-redirect-uri>';
const AUTH_URL = `https://www.dropbox.com/oauth2/authorize?client_id=${CLIENT_ID}&response_type=code&redirect_uri=${encodeURIComponent(REDIRECT_URI)}`;
// Redirect the user to the Dropbox authentication page
window.location.href = AUTH_URL;
After the user grants permission to our app, Dropbox will redirect the user to the REDIRECT_URI
with a code
query parameter. We can then use this code to obtain an access token for the user:
const CLIENT_ID = '<your-client-id>';
const CLIENT_SECRET = '<your-client-secret>';
const REDIRECT_URI = '<your-redirect-uri>';
const CODE = '<code-obtained-from-dropbox>';
// Request an access token from Dropbox
fetch('https://api.dropboxapi.com/oauth2/token', {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: `code=${CODE}&grant_type=authorization_code&client_id=${CLIENT_ID}&client_secret=${CLIENT_SECRET}&redirect_uri=${encodeURIComponent(REDIRECT_URI)}`
})
.then(response => response.json())
.then(data => console.log(data));
Uploading Files to Dropbox
With authentication out of the way, we can start working with the Dropbox API. Let's start by uploading a file to Dropbox using JavaScript:
/**
* Upload a file to Dropbox.
*
* @param {string} accessToken - The user's access token.
* @param {File} file - The file to upload.
* @param {string} path - The path to upload the file to (e.g., "/my-folder/my-file.txt").
*/
async function uploadFile(accessToken, file, path) {
const formData = new FormData();
formData.append('file', file);
const response = await fetch(`https://content.dropboxapi.com/2/files/upload`, {
method: 'POST',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/octet-stream',
'Dropbox-API-Arg': JSON.stringify({ path })
},
body: formData
});
const data = await response.json();
return data;
}
// Example usage:
const accessToken = '<user-access-token>';
const file = new File(['Hello, world!'], 'hello.txt', { type: 'text/plain' });
const path = '/hello.txt';
uploadFile(accessToken, file, path).then(data => console.log(data));
Downloading Files from Dropbox
We can also download files from Dropbox using JavaScript:
/**
* Download a file from Dropbox.
*
* @param {string} accessToken - The user's access token.
* @param {string} path - The path of the file to download (e.g., "/my-folder/my-file.txt").
*/
async function downloadFile(accessToken, path) {
const response = await fetch(`https://content.dropboxapi.com/2/files/download`, {
method: 'POST',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Dropbox-API-Arg': JSON.stringify({ path })
}
});
const data = await response.text();
return data;
}
// Example usage:
const accessToken = '<user-access-token>';
const path = '/hello.txt';
downloadFile(accessToken, path).then(data => console.log(data));
Conclusion
In this blog post, we explored how to work with the Dropbox API in JavaScript. We covered the basics of authentication, uploading files, and downloading files. The Dropbox API provides many other methods as well, so be sure to check out the API documentation for more information.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes