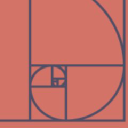
Dummy API
DevelopmentPublic API Docs for https://n161.tech/t/dummyapi/
DummyAPI is a public RESTful API developed for educational purposes by N161. It provides mock data for testing and learning API consumption. Here are some examples of how to use the API with JavaScript.
Retrieving all users
To retrieve all users, send a GET request to the '/users' endpoint.
fetch('https://dummyapi.io/data/api/user',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
Retrieving a single user
To retrieve a single user, send a GET request to the '/user/:userId' endpoint and replace :userId with the desired user ID.
fetch('https://dummyapi.io/data/api/user/123456',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
Retrieving all posts
To retrieve all posts, send a GET request to the '/posts' endpoint.
fetch('https://dummyapi.io/data/api/post',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
Retrieving all posts by a user
To retrieve all posts made by a user, send a GET request to the '/user/:userId/post' endpoint and replace :userId with the desired user ID.
fetch('https://dummyapi.io/data/api/user/123456/post',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
Retrieving all tags
To retrieve all available tags, send a GET request to the '/tag' endpoint.
fetch('https://dummyapi.io/data/api/tag',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
Retrieving all post with tags
To retrieve all posts with a specific tag, send a GET request to the '/tag/:tagName/post' endpoint and replace :tagName with the desired tag name.
fetch('https://dummyapi.io/data/api/tag/sports/post',{
headers: {
'app-id': 'YOUR_APP_ID'
}
})
.then(response => response.json())
.then(data => console.log(data))
That's it! You can use these examples to start interacting with the DummyAPI and learn how to use APIs with JavaScript. Remember to replace YOUR_APP_ID with your own API key.