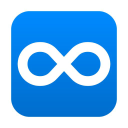
Dynalist
Documents & ProductivityExploring Dynalist's APIs
Dynalist's APIs allow developers to programmatically interact with Dynalist data. In this blog, we will be exploring the documentation for Dynalist's public APIs available at https://apidocs.dynalist.io/ and providing JavaScript examples for each API.
Authentication
To use Dynalist's APIs, you need an API key. You can get your API key by following the instructions given here.
Once you have your API key, you can add it to your script as shown below:
const apiKey = "YOUR_API_KEY";
API Calls
Document API
The Document API allows you to retrieve, create, update, and delete documents. The detailed documentation for the Document API can be found here.
Example: Retrieving Documents
const endpoint = "https://dynalist.io/api/v1/document/list";
const options = {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Token ${apiKey}`
},
body: JSON.stringify({
token: apiKey
})
};
fetch(endpoint, options)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
Node API
The Node API allows you to retrieve, create, update, and delete nodes within a document. The detailed documentation for the Node API can be found here.
Example: Retrieving Nodes
const document_id = "DOCUMENT_ID";
const endpoint = `https://dynalist.io/api/v1/file/list?id=${document_id}`;
const options = {
method: "GET",
headers: {
"Authorization": `Token ${apiKey}`
}
};
fetch(endpoint, options)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
Inbox API
The Inbox API allows you to retrieve, create, and delete items in your Dynalist Inbox. The detailed documentation for the Inbox API can be found here.
Example: Retrieving Inbox Items
const endpoint = "https://dynalist.io/api/v1/inbox/get_all";
const options = {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Token ${apiKey}`
},
body: JSON.stringify({
token: apiKey
})
};
fetch(endpoint, options)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
Conclusion
In this blog, we explored Dynalist's public APIs and provided JavaScript examples for each API. We hope that this will help you get started with using Dynalist's APIs in your projects. Be sure to check out the detailed documentation available at https://apidocs.dynalist.io/ for more information on the APIs and their capabilities.