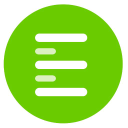
Edamam
Food & DrinkUtilizing the Edamam API with JavaScript
Are you someone who loves to cook and experiment with different recipes? Or perhaps you're a developer who's working on a project that requires information about food and nutrition. Either way, the Edamam API is a great resource to have at your disposal.
The Edamam API provides access to a vast database of food and nutrition information. You can use this API to get nutritional data, search for recipes, and more. In this post, we'll discuss how to use the Edamam API with JavaScript.
Getting Started
First, you'll need to sign up for a developer account to obtain an API key from the Edamam website. Once you have your API key, you can use it to send requests to the Edamam API.
Example Code
To use the Edamam API with JavaScript, we'll need to make API requests using the fetch()
method. Below are examples of how to use some of the Edamam API endpoints.
Recipe Search
const app_id = 'YOUR_APP_ID';
const app_key = 'YOUR_APP_KEY';
const recipe_name = 'chicken';
fetch(`https://api.edamam.com/search?q=${recipe_name}&app_id=${app_id}&app_key=${app_key}`)
.then(response => response.json())
.then(data => console.log(data.hits))
.catch(error => console.error(error));
In the above example, we're searching for recipes that contain the word "chicken". We're using the fetch()
method to make a GET request to the following endpoint: https://api.edamam.com/search?q=${recipe_name}&app_id=${app_id}&app_key=${app_key}
. This endpoint includes the search query, recipe_name
, and the API key and ID. Finally, we're logging the response data to the console.
Nutritional Data
const app_id = 'YOUR_APP_ID';
const app_key = 'YOUR_APP_KEY';
const food = 'apple';
fetch(`https://api.edamam.com/api/food-database/v2/parser?nutrition-type=logging&app_id=${app_id}&app_key=${app_key}&ingr=${food}`)
.then(response => response.json())
.then(data => console.log(data.parsed[0].food.nutrients))
.catch(error => console.error(error));
Here, we're requesting the nutritional data for an apple. We're using the fetch()
method to make a GET request to the following endpoint: https://api.edamam.com/api/food-database/v2/parser?nutrition-type=logging&app_id=${app_id}&app_key=${app_key}&ingr=${food}
. This endpoint includes the API key and ID, and the food item we're searching for. Finally, we're logging the nutrient data for the food item to the console.
Conclusion
The Edamam API is a powerful tool that can be used to obtain food and nutrition information. With JavaScript, we can easily make requests to the Edamam API and retrieve data. By using the examples outlined in this post, you'll be well on your way to integrating the Edamam API into your own projects. Happy cooking!