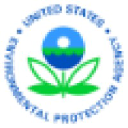
EPA
GovernmentThe US Environmental Protection Agency (EPA) provides web services and datasets that are freely accessible to public. This robust API is designed to allow developers to tap into the vast data sets held by the EPA, which is essential for building applications that relate to environmental issues, such as pollution, climate change, waste management and more. Further information and comprehensive documentation can be found at their official website here.
Using this API offers several benefits including:
- Access to extensive and accurate environmental datasets held by the EPA.
- Facilitates creation of environmentally focused applications.
- Provides regular updates, ensuring you have the most current data.
- Enhances transparency by providing public access to environmental data.
- Fosters innovation by encouraging the creation of environmental solutions.
Here is a simple JavaScript code example that demonstrates how to call this API:
var request = require('request');
var options = {
url: 'https://api.data.gov/epa/your-end-point/',
headers: {
'X-API-KEY': 'Your-API-Key'
}
};
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
var info = JSON.parse(body);
console.log(info);
}
}
request(options, callback);
Please ensure to replace 'your-end-point' with the correct endpoint that you want to access, and 'Your-API-Key' with your actual API key.