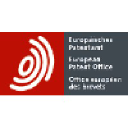
EPO
PatentThe European Patent Search System API offers a comprehensive solution for accessing a vast database of European patent information. By leveraging this API, developers can integrate powerful search capabilities directly into their applications, enabling users to efficiently search for patents across various categories and jurisdictions. With a well-documented interface available at developers.epo.org, this API stands out as an essential tool for researchers, inventors, and businesses looking to stay informed about patent developments in Europe. The robust capabilities of the API ensure users can find detailed patent data, including abstracts, classifications, and legal status, streamlining the research process significantly.
Utilizing the European Patent Search System API comes with a range of benefits. Key advantages include real-time access to an extensive database of patents, the ability to perform complex queries with multiple parameters, support for various programming languages, efficient data retrieval, and integration capabilities that enhance user experience. These features make the API not only a valuable resource for individual researchers but also an essential asset for organizations operating in innovation-driven industries.
- Real-time access to an extensive database of patents
- Ability to perform complex queries with multiple parameters
- Support for various programming languages
- Efficient data retrieval processes
- Enhanced user experience through seamless integration
Here’s a simple JavaScript code example demonstrating how to call the European Patent Search System API:
const fetch = require('node-fetch');
async function searchPatents(query) {
const url = `https://ops.epo.org/3.2/rest-services/published-data/search?q=${encodeURIComponent(query)}`;
const response = await fetch(url, {
method: 'GET',
headers: {
'Accept': 'application/json',
'Authorization': 'Bearer YOUR_ACCESS_TOKEN' // Optional authorization, if required
}
});
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
const data = await response.json();
console.log(data);
}
// Example usage
searchPatents('renewable energy');