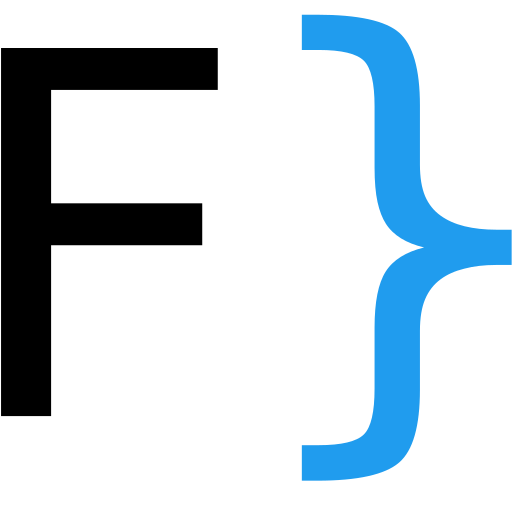
FakeJSON
DevelopmentIntroduction to FakeJSON public API
FakeJSON is a public API that provides mock JSON data for testing purposes. This API can be used for generating fake data for various applications such as web and mobile development. In this article, we will discuss various API examples in JavaScript that can be used to fetch fake data from the FakeJSON API.
Getting Started
To use the FakeJSON API, you need to obtain an API key. You can register a new account on FakeJSON and get the API key by following the instructions on their website. Once you have the API key, you can use it to make API requests.
API Examples
Example 1: Generating Fake Data for Users
fetch('https://app.fakejson.com/q', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <YOUR_API_KEY>'
},
body: JSON.stringify({
token: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
data: {
firstName: '{{firstName}}',
lastName: '{{lastName}}',
email: '{{email}}',
phone: '{{phone}}',
street: '{{streetAddress}}',
city: '{{city}}',
state: '{{stateAbbr}}',
country: '{{country}}',
zip: '{{zipCode}}'
}
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are using the fetch() method to make a POST request to the FakeJSON API. We are passing our API key in the Authorization header and a JSON payload containing the data we want to generate. This will return a JSON object containing the fake data for the requested fields.
Example 2: Generating Fake Data for Products
fetch('https://app.fakejson.com/q', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <YOUR_API_KEY>'
},
body: JSON.stringify({
token: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
data: {
productCode: '{{productCode}}',
productName: '{{productName}}',
productDescription: '{{loremIpsum}}',
productPrice: '{{numberLength 2}}.{{numberLength 2}}'
}
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are generating fake product data. We are passing the fields we want to generate in the data object. This will return a JSON object containing the fake product data.
Example 3: Generating Fake Data for Images
fetch('https://app.fakejson.com/q', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <YOUR_API_KEY>'
},
body: JSON.stringify({
token: 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx',
data: {
imageUrl: '{{imageUrl 300 300 animals}}',
imageDescription: '{{catchPhrase}}'
}
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error))
In this example, we are generating fake image data. We are passing the fields we want to generate in the data object. This will return a JSON object containing the fake image data.
Conclusion
FakeJSON provides a simple and easy-to-use API for generating fake data for testing purposes. In this article, we have discussed various API examples in JavaScript that can be used to fetch fake data from the FakeJSON API. These examples can be easily modified to generate custom data for your applications.