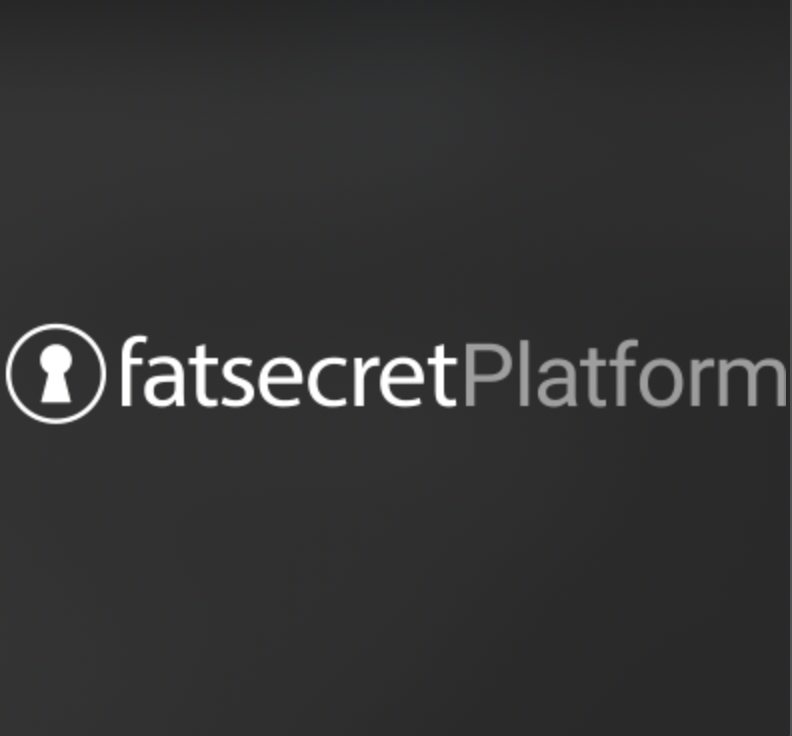
FatSecret Platform API
Food & DrinkThe #1 food and nutrition database in the world, utilized by more than 10,000 developers, in more than 50 countries contributing in excess of 500 million API calls every month. Complete access to a comprehensive database of accurate food and nutrition information as well as exercise and weight management tools.
📚 Documentation & Examples
Everything you need to integrate with FatSecret Platform API
🚀 Quick Start Examples
// FatSecret Platform API API Example
const response = await fetch('https://platform.fatsecret.com/api/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Working with the FatSecret API
If you are looking for a food and nutrition database API, the FatSecret platform is worth exploring. The FatSecret API provides a wide variety of endpoints allowing developers to incorporate a wealth of food and nutrition data into their applications.
Getting Started
Before we dive into the examples, we need to make sure that we have an API key. You can get your API key by navigating to the FatSecret Developer Center.
Once you have your API key, you can start making requests.
API Endpoints and Example Code
Search Food
To search for food, you can use the food.search
API endpoint. Here's an example of how you can search for "apple":
const axios = require('axios');
const appId = 'YOUR_APP_ID';
const appKey = 'YOUR_APP_KEY';
const query = 'apple';
axios.get(`https://platform.fatsecret.com/rest/server.api?` +
`method=food.search&` +
`search_expression=${query}&` +
`format=json&` +
`oauth_consumer_key=${appId}&` +
`oauth_signature_method=HMAC-SHA1&` +
`oauth_timestamp=${Math.floor(Date.now() / 1000)}&` +
`oauth_nonce=${Math.floor(Math.random() * 1000000000)}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error.response.data);
});
Get Food Information
To get information about a specific food item, you can use the food.get
API endpoint. Here's an example code that retrieves the information for the item with food_id
of 41000454
:
const axios = require('axios');
const appId = 'YOUR_APP_ID';
const appKey = 'YOUR_APP_KEY';
const foodId = '41000454';
axios.get(`https://platform.fatsecret.com/rest/server.api?` +
`method=food.get&` +
`food_id=${foodId}&` +
`format=json&` +
`oauth_consumer_key=${appId}&` +
`oauth_signature_method=HMAC-SHA1&` +
`oauth_timestamp=${Math.floor(Date.now() / 1000)}&` +
`oauth_nonce=${Math.floor(Math.random() * 1000000000)}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error.response.data);
});
Get Recipe Information
If you want recipe information, you can use the recipe.get
API endpoint. This example retrieves the information for the recipe with recipe_id
of 6130071874
:
const axios = require('axios');
const appId = 'YOUR_APP_ID';
const appKey = 'YOUR_APP_KEY';
const recipeId = '6130071874';
axios.get(`https://platform.fatsecret.com/rest/server.api?` +
`method=recipe.get&` +
`recipe_id=${recipeId}&` +
`format=json&` +
`oauth_consumer_key=${appId}&` +
`oauth_signature_method=HMAC-SHA1&` +
`oauth_timestamp=${Math.floor(Date.now() / 1000)}&` +
`oauth_nonce=${Math.floor(Math.random() * 1000000000)}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error.response.data);
});
Get Recipe Information with Detailed Nutritional Information
If you want more detailed nutritional information for a recipe, you can use the recipe.get
, but you need to set the show_nutrition
parameter to 1
.
const axios = require('axios');
const appId = 'YOUR_APP_ID';
const appKey = 'YOUR_APP_KEY';
const recipeId = '6130071874';
axios.get(`https://platform.fatsecret.com/rest/server.api?` +
`method=recipe.get&` +
`recipe_id=${recipeId}&` +
`format=json&` +
`show_nutrition=1&` +
`oauth_consumer_key=${appId}&` +
`oauth_signature_method=HMAC-SHA1&` +
`oauth_timestamp=${Math.floor(Date.now() / 1000)}&` +
`oauth_nonce=${Math.floor(Math.random() * 1000000000)}`)
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error.response.data);
});
Conclusion
In this short guide, we showed how to access the FatSecret API and provided several examples of how to retrieve food and recipe information. With this information, you can build a variety of applications, including recipe managers, meal planners, and nutrition trackers. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes