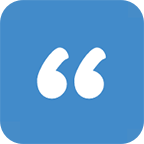
FavQs.com
PersonalityUsing the Public API of FavQs
FavQs is a quotes-sharing platform that offers a public API that allows developers to access quotes data. In this tutorial, we will explore how to work with this API by using JavaScript. Let's get started!
Getting Started
To use the public API of FavQs, you need to obtain an API key, which you can get by signing up on their website (https://favqs.com/). Once you have your API key, you can use it to make requests to the API.
API Endpoints
The FavQs API has several endpoints that you can use to get quotes data. Here are some of the endpoints:
/api/qotd
: returns the quote of the day/api/quotes
: returns a list of quotes/api/quotes/:id
: returns a specific quote by its ID
Making Requests
To make requests to the API, we will be using the fetch()
method of JavaScript. Here's an example of how to make a request to get the quote of the day:
const apiKey = 'YOUR_API_KEY';
const endpoint = 'https://favqs.com/api/qotd';
fetch(endpoint, {
headers: {
Authorization: `Token token=${apiKey}`,
},
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we first define the apiKey
and endpoint
variables. We then use the fetch()
method to make a GET request to the API endpoint. We pass our API key as an authorization header in the request. Finally, we parse the response as JSON and log it to the console.
Conclusion
In this tutorial, we learned how to work with the public API of FavQs using JavaScript. We explored the API endpoints and saw how to make requests to them. With this knowledge, you can now create your own applications that use quotes data from FavQs.