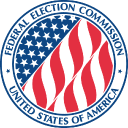
FEC
GovernmentExploring the FEC API with JavaScript
The Federal Election Commission (FEC) provides a public API that allows developers to access data related to campaign finance. In this blog post, we will explore the FEC API using JavaScript, and see how we can use it to obtain useful information.
Getting Started
Before we dive into the examples, let's first visit the FEC API developers page and get ourselves an API key. Once you have obtained the key, you can start making requests to the API.
The FEC API endpoint structure is as follows:
https://api.open.fec.gov/v1/{method_name}?api_key={api_key}
Where {method_name}
is the name of the API method you want to call, and {api_key}
is the API key that you obtained earlier. Let's start with a simple example.
Example 1: Retrieving basic information
In this example, we will retrieve basic information about a candidate. Let's retrieve the name of Donald Trump, using the Candidate
method.
const API_KEY = 'YOUR_API_KEY_HERE';
const endpoint = 'https://api.open.fec.gov/v1/candidates/?name=trump&api_key=' + API_KEY;
fetch(endpoint)
.then(response => response.json())
.then(data => {
const results = data.results;
console.log(results[0].name);
});
In this example, we made a request to the Candidate
method, passing in the name "trump" as a parameter. We used the fetch
method to make the request, and then parsed the JSON response to obtain the name of the candidate.
Example 2: Filtering results
In this next example, we will filter the results of our query to retrieve only results that meet certain criteria. Let's retrieve the names of all candidates whose party affiliation is "DEM", by using the Candidate
method and filtering by party.
const API_KEY = 'YOUR_API_KEY_HERE';
const endpoint = 'https://api.open.fec.gov/v1/candidates/?party=DEM&api_key=' + API_KEY;
fetch(endpoint)
.then(response => response.json())
.then(data => {
const results = data.results;
const names = results.map(candidate => candidate.name);
console.log(names);
});
In this example, we added the parameter party=DEM
to the URL of our request, which filters out all candidates whose party affiliation is not "DEM". We then used the map
method to extract just the names of the candidates from the results.
Example 3: Advanced filtering
In this final example, we will combine multiple filters to obtain more granular results. Let's retrieve the names of all candidates whose party affiliation is "REP" and whose total receipts is greater than $10,000, using the Candidate
and Totals
methods.
const API_KEY = 'YOUR_API_KEY_HERE';
const endpoint = 'https://api.open.fec.gov/v1/candidates/?api_key=' + API_KEY +
'&party=REP' +
'&totals.has_total_receipts=true' +
'&sort_null_only=false' +
'&sort_nulls_last=false' +
'&sort=-totals.total_receipts';
fetch(endpoint)
.then(response => response.json())
.then(data => {
const results = data.results;
const names = results.map(candidate => candidate.name);
console.log(names);
});
In this example, we used multiple parameters to filter our results. We used the party
parameter to filter by party affiliation, and the totals.has_total_receipts
parameter to filter by candidates who have total receipts. We also used the sort
parameter to sort our results by total receipts in descending order.
Conclusion
In this blog post, we explored the FEC API using JavaScript. We covered the basics of making requests to the API, filtering results, and using multiple parameters to obtain more granular results. With these examples, you should have the knowledge to start exploring the FEC API and retrieving the data you need.