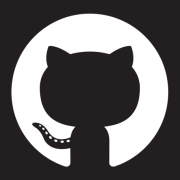
Feedi
DevelopmentIntroduction to Feedi API
Feedi API is a public API that provides access to multiple news sources from around the world. With the Feedi API, developers can access news and articles from various websites and sources, including CNN, BBC, The Guardian, and more. In this blog post, we'll walk through some of the basic features and API calls for Feedi, including sample code in JavaScript.
Getting Started
To get started, you'll need to sign up for the Feedi API and obtain an API key. You can sign up at the API website. Once you have your API key, you can start making API calls.
API Endpoints
The Feedi API has several endpoints that allow developers to access news and articles from various sources. Here are some of the available endpoints:
Get Top Headlines
This endpoint provides the top headlines from various news sources. Here's the sample code to retrieve the top headlines using JavaScript:
const apiKey = 'your_api_key_here';
const url = `https://api.feedi.net/v1/top-headlines?apiKey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Get News from a Specific Source
This endpoint allows you to retrieve news from a specific news source. Here's the sample code to retrieve news from CNN using JavaScript:
const apiKey = 'your_api_key_here';
const url = `https://api.feedi.net/v1/sources/cnn?apiKey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Search for News
This endpoint allows you to search for news based on a keyword. Here's the sample code to search for news related to "technology" using JavaScript:
const apiKey = 'your_api_key_here';
const keyword = 'technology';
const url = `https://api.feedi.net/v1/search?q=${keyword}&apiKey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
In this blog post, we covered some of the basic features and API calls for the Feedi API. With these API endpoints, developers can easily access news and articles from various sources. The sample code in JavaScript can be used as a starting point for building applications that use the Feedi API.