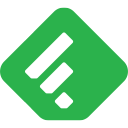
Feedly API
Documents & ProductivityExploring the Feedly Developer API
Feedly is one of the most popular RSS aggregators out there, and with its developer API, users and developers alike can tap into the power of Feedly's content organizing capabilities. In this blog post, we'll go over everything you need to know to get started with the Feedly API, including example code in JavaScript.
What is the Feedly API?
The Feedly API is a developer API that allows users to interact with their Feedly feeds. With the API, you can build integrations into your own applications to access specific feeds and get updates on them in real-time.
Getting started with the Feedly API
To get started with the Feedly API, you will need to create an account, which you can do at the Feedly developer website. Once you have an account, you can then access the API endpoints to build your integrations.
Example code in JavaScript
Here are some example code snippets in JavaScript that are commonly used with the Feedly API:
Retrieving a user's feeds
const request = require('request');
const FEEDLY_API_URL = 'https://cloud.feedly.com/v3';
function getUserFeeds(token, callback) {
const options = {
url: `${FEEDLY_API_URL}/collections`,
headers: {
Authorization: `Bearer ${token}`
}
};
request.get(options, (error, response) => {
if (error) {
console.log(error);
return;
}
const feeds = JSON.parse(response.body);
callback(feeds);
});
}
Retrieving the latest articles from a feed
const request = require('request');
const FEEDLY_API_URL = 'https://cloud.feedly.com/v3';
function getFeedArticles(token, feedId, callback) {
const options = {
url: `${FEEDLY_API_URL}/streams/contents?streamId=${feedId}&count=10`,
headers: {
Authorization: `Bearer ${token}`
}
};
request.get(options, (error, response) => {
if (error) {
console.log(error);
return;
}
const articles = JSON.parse(response.body);
callback(articles);
});
}
Adding an article to a user's feed
const request = require('request');
const FEEDLY_API_URL = 'https://cloud.feedly.com/v3';
function addArticleToFeed(token, feedId, article) {
const options = {
url: `${FEEDLY_API_URL}/entries`,
headers: {
Authorization: `Bearer ${token}`,
'Content-Type': 'application/json'
},
json: {
origin: {
streamId: feedId,
title: article.title,
htmlUrl: article.url
},
title: article.title,
categories: [
{
label: 'Saved',
id: 'user/saved'
}
]
}
};
request.post(options, (error, response) => {
if (error) {
console.log(error);
return;
}
console.log(response.body);
});
}
Conclusion
If you're looking to integrate Feedly's capabilities into your own applications, the Feedly API is a great place to start. With the example code in JavaScript that we've provided, you have a solid foundation for building your integration. Give it a try and see how you can enhance your users' experience with a powerful RSS aggregator like Feedly!