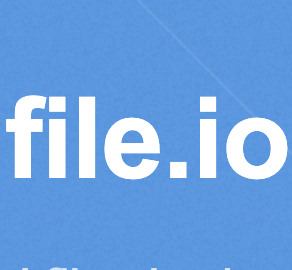
File.io
Documents & ProductivityUsing File.io Public API with JavaScript
File.io is a simple file sharing and storage platform that provides a public API. In this blog post, we will explore how to use File.io Public API with JavaScript.
Prerequisites
Before we start, you need to have the following:
- A text editor or an IDE (e.g., Visual Studio Code, Sublime Text, or Atom)
- Basic knowledge of JavaScript
Getting Started
To use File.io Public API with JavaScript, we need to make an HTTP request to the API endpoint. We can do this with the fetch
method, which is a native JavaScript function that sends a request to a URL and returns a Promise.
Uploading a File
To upload a file, we need to make a POST request to the File.io API endpoint with the file data in the request body. The API returns a JSON object containing the file metadata, including the download URL. Here's an example of uploading a file using JavaScript:
// File to upload as a binary string
const fileData = '0123456789ABCDEF';
// Set API endpoint URL
const apiUrl = 'https://file.io';
// Make POST request to API endpoint
fetch(apiUrl, {
method: 'POST',
body: fileData
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Downloading a File
To download a file, we need to make a GET request to the URL provided by the File.io API endpoint. The URL expires after a certain amount of time, so we need to download the file immediately after receiving the URL. Here's an example of downloading a file using JavaScript:
// File URL to download
const fileUrl = 'https://file.io/WdYTUJ';
// Make GET request to file URL
fetch(fileUrl)
.then(response => response.blob())
.then(blob => {
const url = URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'filename.txt';
a.click();
URL.revokeObjectURL(url);
})
.catch(error => console.error(error));
Conclusion
Using the File.io Public API with JavaScript allows us to easily upload and download files programmatically. With the fetch
method, we can make HTTP requests to the API endpoint and manipulate the response data as needed. In this blog post, we've demonstrated how to use the API to upload and download files using JavaScript. We hope this serves as a useful guide for anyone wanting to integrate the File.io API into their JavaScript application.