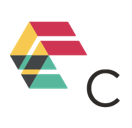
Flight Stats API
TransportationFlightStats Weather API
The FlightStats Weather API provides weather information for airports and regions around the world. This API can be used to retrieve information such as current conditions, temperature, precipitation, wind speed and direction, and more.
Authentication
The FlightStats Weather API requires authentication using an API key. You can obtain an API key by signing up for a free account on the FlightStats website.
Endpoints
There are two endpoints available in the FlightStats Weather API:
- /weather/forecast - Returns a weather forecast for a specific airport or region.
- /weather/current - Returns the current weather conditions for a specific airport.
API Examples in JavaScript
Here are some example API calls using JavaScript:
1. Retrieve a weather forecast for a specific airport
const apiKey = 'YOUR_API_KEY';
const airportCode = 'SFO';
fetch(`https://api.flightstats.com/flex/weather/rest/v1/json/forecast/${airportCode}?appId=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log('Weather forecast:', data);
})
.catch(error => console.error(error));
2. Retrieve the current weather conditions for a specific airport
const apiKey = 'YOUR_API_KEY';
const airportCode = 'SFO';
fetch(`https://api.flightstats.com/flex/weather/rest/v1/json/current/${airportCode}?appId=${apiKey}`)
.then(response => response.json())
.then(data => {
console.log('Current weather conditions:', data);
})
.catch(error => console.error(error));
Note that in these examples, YOUR_API_KEY
should be replaced with your FlightStats API key, and SFO
should be replaced with the airport code for the airport you are interested in.
Conclusion
The FlightStats Weather API is a useful tool for retrieving weather information for airports and regions around the world. With a little bit of JavaScript code, you can easily retrieve weather forecasts and current weather conditions.