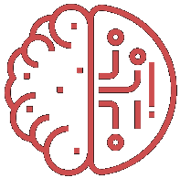
Football Prediction
Sports & FitnessBoggio Analytics FP API
Boggio Analytics provides a public API (Application Programming Interface) that enables developers to access its fingerprinting technology. This technology allows for the detection of unique attributes of a device, such as browser, operating system, and plugins, without the use of cookies. The user data is processed entirely client-side, and no personal information is stored or transmitted.
The FP API uses JavaScript, and developers have the option to make requests either synchronously or asynchronously. Below are some examples of the API calls that can be made in JavaScript:
Getting the fingerprint of a device
// Synchronous request
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://boggio-analytics.com/fp-api/fingerprint", false);
xhr.send(null);
console.log(xhr.responseText);
// Asynchronous request
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://boggio-analytics.com/fp-api/fingerprint", true);
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
console.log(xhr.responseText);
}
}
xhr.send();
Setting custom user IDs
// Synchronous request
var xhr = new XMLHttpRequest();
xhr.open("POST", "https://boggio-analytics.com/fp-api/user-id", false);
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.send(JSON.stringify({"userId": "custom123"}));
console.log(xhr.responseText);
// Asynchronous request
var xhr = new XMLHttpRequest();
xhr.open("POST", "https://boggio-analytics.com/fp-api/user-id", true);
xhr.setRequestHeader("Content-Type", "application/json;charset=UTF-8");
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
console.log(xhr.responseText);
}
}
xhr.send(JSON.stringify({"userId": "custom123"}));
Getting user profile data
// Synchronous request
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://boggio-analytics.com/fp-api/user-profile", false);
xhr.send(null);
console.log(xhr.responseText);
// Asynchronous request
var xhr = new XMLHttpRequest();
xhr.open("GET", "https://boggio-analytics.com/fp-api/user-profile", true);
xhr.onreadystatechange = function() {
if (xhr.readyState == 4 && xhr.status == 200) {
console.log(xhr.responseText);
}
}
xhr.send();
Note that some of the API calls require an API key. To obtain an API key, sign up for an account on the Boggio Analytics website and follow the instructions.
In conclusion, the Boggio Analytics FP API provides developers with a powerful tool for obtaining device fingerprint data. With the examples provided, developers can easily integrate this technology into their own projects.