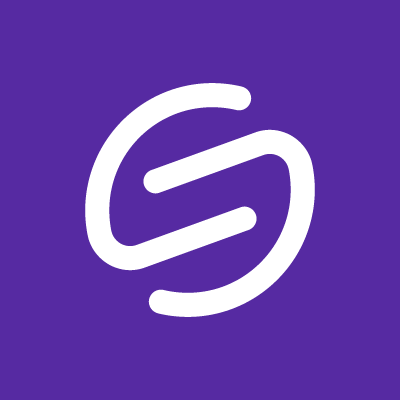
Fromsmash
Cloud Storage & File SharingFromsmash Public API Docs
Fromsmash is a file-sharing service that allows users to easily share large files with others. The Fromsmash public API provides developers with a way to programmatically interact with the service, enabling them to integrate file-sharing capabilities into their applications. In this blog post, we'll explore the Fromsmash public API docs and provide some example code in JavaScript.
Example API Requests
Before we dive into the code, let's take a quick look at some example API requests. All Fromsmash API requests are sent via HTTPS to https://api.fromsmash.com/
. Here are a few example requests:
Upload a File
To upload a file to Fromsmash, you must first generate a unique URL using the /upload_url
endpoint. Then, you can use the provided URL to upload your file. Here's an example:
const fetch = require('node-fetch');
const fs = require('fs');
const filepath = '/path/to/my/file.pdf';
const filename = 'file.pdf';
fetch('https://api.fromsmash.com/upload_url', {
method: 'POST',
body: JSON.stringify({ name: filename })
})
.then(response => response.json())
.then(data => {
const upload_url = data.upload_url;
const file = fs.createReadStream(filepath);
fetch(upload_url, {
method: 'PUT',
body: file
});
});
List Files
To list all files uploaded to your Fromsmash account, use the /files
endpoint. Here's an example:
const fetch = require('node-fetch');
fetch('https://api.fromsmash.com/files')
.then(response => response.json())
.then(data => console.log(data));
Download a File
To download a file from Fromsmash, use the /download
endpoint. Here's an example:
const fetch = require('node-fetch');
const file_id = 'abc123';
fetch(`https://api.fromsmash.com/download/${file_id}`)
.then(response => {
if (response.ok) {
const filename = response.headers.get('content-disposition').split('=')[1];
const file = fs.createWriteStream(`/path/to/my/downloads/${filename}`);
response.body.pipe(file);
} else {
console.log(`Error downloading file with id ${file_id}`);
}
});
Conclusion
In this blog post, we've explored the Fromsmash public API docs and provided some example code in JavaScript. With these tools, developers can easily integrate file-sharing capabilities into their applications and provide their users with a seamless file-sharing experience.