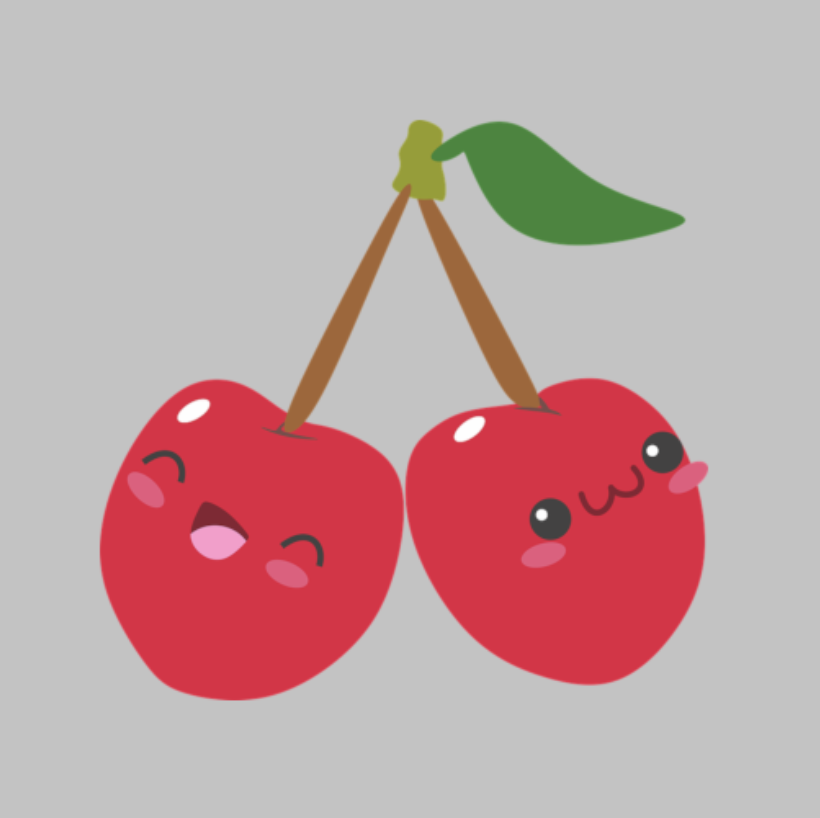
FruityVice
Food & DrinkA powerful webservice which provides data for all kinds of fruit! You can use Fruityvice to find out interesting information about fruit and educate yourself. This web service is completely free to use and contribute to. With Fruityvice you can receive interesting data from any fruit of your choosing. On top of that you can add fruits by yourself as well! Added fruits will first have to be approved by an admin to avoid any errors in the data. The shown data is based on 100 grams of the listed fruit. The owner does not guarantee the available data is 100% flawless, however he will do his best to fix any wrong data.
📚 Documentation & Examples
Everything you need to integrate with FruityVice
🚀 Quick Start Examples
// FruityVice API Example
const response = await fetch('https://www.fruityvice.com', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Accessing Fruityvice Public API Using JavaScript
If you're looking for a comprehensive API that provides information on fruits and their nutritional value, then Fruityvice's public API is the perfect choice for you. With API endpoints that provide data on fruit images, nutritional information, and even random fruit facts, Fruityvice has everything you need to create a robust fruit-based application.
Getting Started
To access Fruityvice's public API, you'll need to sign up for an API key on their website. Once you have your API key, you're ready to start making requests.
API Endpoints
Fruityvice offers several API endpoints that can be accessed using JavaScript. Here are some of the most useful endpoints:
Get All Fruits
To retrieve details about all the fruits available in the Fruityvice database, use the following code:
fetch('https://www.fruityvice.com/api/fruit/all')
.then(response => response.json())
.then(data => console.log(data));
This will return an array of objects containing the details of each fruit, including its name, image, and nutritional information.
Get Fruit Details By Name
To retrieve the details of a specific fruit based on its name, use the following code:
fetch('https://www.fruityvice.com/api/fruit/[fruit-name]')
.then(response => response.json())
.then(data => console.log(data));
Replace [fruit-name]
with the name of the fruit you're interested in. This will return an object containing the details of the specified fruit.
Get Random Fruit
To retrieve details of a random fruit from the Fruityvice database, use the following code:
fetch('https://www.fruityvice.com/api/fruit/random')
.then(response => response.json())
.then(data => console.log(data));
This will return an object containing the details of a random fruit.
Get Fruit Image
To retrieve the image of a specific fruit based on its name, use the following code:
fetch('https://www.fruityvice.com/api/fruit/[fruit-name]/image')
.then(response => response.json())
.then(data => console.log(data));
Replace [fruit-name]
with the name of the fruit you're interested in. This will return an object containing the image of the specified fruit.
Get Fruit Facts
To retrieve a random fact about a specific fruit based on its name, use the following code:
fetch('https://www.fruityvice.com/api/fruit/[fruit-name]/fun-facts')
.then(response => response.json())
.then(data => console.log(data));
Replace [fruit-name]
with the name of the fruit you're interested in. This will return an object containing a random fact about the specified fruit.
Conclusion
Fruityvice's public API is a great resource for developers creating applications related to fruits and their nutritional value. By using JavaScript to access the API endpoints, you can make requests for information that can be used to create engaging and informative applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes