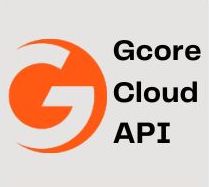
Gcore Cloud
DevelopmentThe Gcore Cloud API is a platform that enables developers to manage their cloud resources and services through a simple and intuitive interface. It provides a wide range of tools and features that allow users to easily deploy, monitor, and scale their cloud applications. With Gcore Cloud API, developers can automate the entire cloud infrastructure, reducing the need for manual intervention and enhancing productivity. The platform is highly customizable, supporting multiple programming languages and integrations with popular cloud providers. Gcore Cloud API is a reliable and efficient solution for organizations looking to optimize their cloud operations and improve their overall performance.
π Documentation & Examples
Everything you need to integrate with Gcore Cloud
π Quick Start Examples
// Gcore Cloud API Example
const response = await fetch('https://docs.gcore.com/cloud', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
A Guide to Using the GCore Cloud API
The GCore Cloud API is a powerful tool for developers who need to integrate cloud-based data storage and retrieval into their applications. This API provides easy-to-use methods for accessing data stored in the cloud through a simple RESTful interface. In this guide, we will explore how to use the GCore Cloud API by providing examples of how to access the API with JavaScript.
Authentication
Before you can start using the GCore Cloud API, you need to authenticate your API calls. This is done by including a valid API key in your request header. You can retrieve your API key by logging into your GCore Cloud account and navigating to the API Keys section.
const apiKey = 'YOUR_API_KEY';
const headers = { 'Authorization': `Bearer ${apiKey}` };
Creating a container
A container in the GCore Cloud API is a location where you can store files and data. To create a container, you need to make a POST request to the /containers
endpoint. Here's an example of how to create a container with JavaScript:
const containerName = 'my-new-container';
const options = {
method: 'POST',
headers: headers,
body: JSON.stringify({ name: containerName })
};
fetch('https://api.gcore.com/cloud/containers', options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Uploading a file
Once you have created a container, you can start uploading files to it. To upload a file, you need to make a POST request to the /upload
endpoint. Here's an example of how to upload a file with JavaScript:
const fileInput = document.querySelector('input[type="file"]');
const file = fileInput.files[0];
const formData = new FormData();
formData.append('file', file);
const options = {
method: 'POST',
headers: headers,
body: formData
};
fetch('https://api.gcore.com/cloud/upload/my-new-container', options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Downloading a file
To download a file from the GCore Cloud API, you need to make a GET request to the /download
endpoint. Here's an example of how to download a file with JavaScript:
const fileId = 'FILE_ID';
const options = {
method: 'GET',
headers: headers
};
fetch(`https://api.gcore.com/cloud/download/my-new-container/${fileId}`, options)
.then(response => response.blob())
.then(blob => console.log(blob))
.catch(error => console.error(error));
Conclusion
The GCore Cloud API provides a comprehensive set of tools for storing and retrieving data in the cloud. By using the examples above, you can get started with the API and start building powerful cloud-based applications.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes