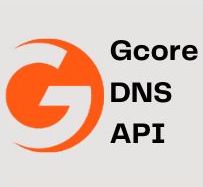
Gcore DNS
DevelopmentExploring the GCore DNS API with JavaScript
Are you looking for a reliable DNS API to use in your web applications? Look no further than GCore DNS API. With its easy-to-use documentation and powerful features, you can start integrating DNS management into your applications in no time!
Getting Started
To get started with the GCore DNS API, you will first need to sign up for an account. Once you have an account, you can start using the API by making requests to the endpoint using JavaScript.
Make sure to use your unique secret API key in each request.
const api_key = "your_secret_api_key";
Examples
List all domains
To list all domains associated with your account, simply make a GET request to the /domains endpoint.
fetch("https://api.gcore.com/dns/v1/domains", {
headers: {
"Authorization": `Bearer ${api_key}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Create a new domain
To create a new domain, make a POST request to the /domains endpoint with a JSON payload containing the domain name.
fetch("https://api.gcore.com/dns/v1/domains", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${api_key}`
},
body: JSON.stringify({ name: "example.com" })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Update a domain
To update a domain, make a PUT request to the /domains/:id endpoint with a JSON payload containing the updated information.
fetch("https://api.gcore.com/dns/v1/domains/123456", {
method: "PUT",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${api_key}`
},
body: JSON.stringify({ name: "new-example.com" })
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Delete a domain
To delete a domain, make a DELETE request to the /domains/:id endpoint.
fetch("https://api.gcore.com/dns/v1/domains/123456", {
method: "DELETE",
headers: {
"Authorization": `Bearer ${api_key}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
With the power of GCore DNS API and the simplicity of JavaScript, managing your DNS has never been easier. Try out some of these examples to see how you can integrate DNS management into your web applications!