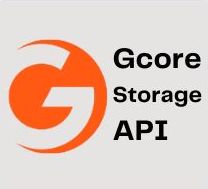
Gcore Storage
Cloud Storage & File SharingWorking with GCore Storage API using JavaScript
GCore Storage API provides developers with the ability to read, write and delete files programmatically. In this blog post, we will explore how to use the Storage API in JavaScript.
Prerequisites
To use the GCore Storage API, you need to create a GCore account and obtain an API key. Once you have your API key, you can authenticate your requests to the API.
API endpoints
The Storage API provides the following endpoints:
/file
- to upload and download files./ls
- to list files and directories./mkdir
- to create a new directory./rm
- to remove a file or directory.
Uploading a file
To upload a file using JavaScript, you can use the Fetch API. Here's an example code snippet:
const apiKey = 'YOUR_API_KEY';
const url = 'https://geo-storage.storage.yandexcloud.net/file/folder/filename';
const file = new File(['content'], 'filename.txt', { type: 'text/plain' });
fetch(url, {
method: 'PUT',
headers: {
'Authorization': apiKey,
'Content-Type': file.type
},
body: file
})
.then(response => response.json())
.then(data => console.log(data));
In this code snippet, we first define our API key and the URL of the file we want to upload. We then create a new File
object with the content we want to upload, and use the Fetch API
to send the PUT
request.
Downloading a file
To download a file using JavaScript, we can use the Fetch API
again. Here's an example code snippet:
const apiKey = 'YOUR_API_KEY';
const url = 'https://geo-storage.storage.yandexcloud.net/file/folder/filename';
fetch(url, {
method: 'GET',
headers: {
'Authorization': apiKey
}
})
.then(response => response.blob())
.then(blob => {
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = 'filename';
a.click();
});
In this code snippet, we first define our API key and the URL of the file we want to download. We then use the Fetch API
to send the GET
request and retrieve the file as a Blob
. We then create a download link and simulate a click()
event to download the file.
Listing files and directories
To list files and directories using JavaScript, we can use the Fetch API
again. Here's an example code snippet:
const apiKey = 'YOUR_API_KEY';
const url = 'https://geo-storage.storage.yandexcloud.net/ls/folder';
fetch(url, {
headers: {
'Authorization': apiKey
}
})
.then(response => response.json())
.then(data => console.log(data));
In this code snippet, we first define our API key and the URL of the directory we want to list. We then use the Fetch API
to send the GET
request and retrieve the list of files and directories.
Creating a new directory
To create a new directory using JavaScript, we can use the Fetch API
again. Here's an example code snippet:
const apiKey = 'YOUR_API_KEY';
const url = 'https://geo-storage.storage.yandexcloud.net/mkdir/folder/new-folder';
fetch(url, {
method: 'POST',
headers: {
'Authorization': apiKey
}
})
.then(response => response.json())
.then(data => console.log(data));
In this code snippet, we first define our API key and the URL of the new directory we want to create. We then use the Fetch API
to send the POST
request.
Removing a file or directory
To remove a file or directory using JavaScript, we can use the Fetch API
again. Here's an example code snippet:
const apiKey = 'YOUR_API_KEY';
const url = 'https://geo-storage.storage.yandexcloud.net/rm/folder/filename';
fetch(url, {
method: 'DELETE',
headers: {
'Authorization': apiKey
}
})
.then(response => response.json())
.then(data => console.log(data));
In this code snippet, we first define our API key and the URL of the file or directory we want to remove. We then use the Fetch API
to send the DELETE
request.
Conclusion
In this blog post, we explored how to use the GCore Storage API in JavaScript. We covered uploading, downloading, listing, creating and removing files and directories using the Fetch API
. With this knowledge, you can integrate the Storage API in your web applications and automate your file management tasks.